How To Setup JUnit Environment For Your First Test
Sri Priya
Posted On: July 31, 2024
337449 Views
18 Min Read
JUnit is a widely used unit testing framework. When combined with Selenium, it enhances the web application testing process. JUnit utilizes annotations to identify test methods and supports various assertions, test suites, and test maintenance.
To start implementing JUnit on your machine, it’s important to understand the basics of JUnit and its architecture. Additionally, you will need to know how to setup JUnit from scratch.
TABLE OF CONTENTS
What Is JUnit?
JUnit is a popular framework for automating unit testing in Java. It follows the principle of “Testing first, then coding,” which means you write tests before implementing the code that the tests will validate.
With this framework, you create test cases to perform unit testing. A unit test case is a block of code that verifies whether the program logic is functioning as expected.
Here are the reasons why JUnit is important for unit testing:
- Improves Code Quality: It helps make code more readable, stable, and error-free.
- Early Bug Detection: It helps identify bugs early in development, increasing application stability.
- Annotations and Assertions: It provides annotations, test methods, hooks, and assertions to verify expected results.
- Test Runners: It includes test runners to execute tests and report results.
- Automated Testing: Its tests run automatically and provide feedback on whether the code behaves as expected. Test results are often displayed in a progress bar or similar visual format.
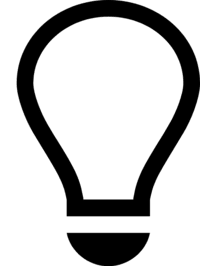
Run web app testing using the JUnit framework with over 3000+ browser and OS combinations. Try LambdaTest Today!
Now that you understand the basics of JUnit and its importance for unit testing let’s learn about its working architecture.
JUnit 5 Architecture
JUnit 5 is organized into several modules, divided across three distinct sub-projects, each serving a specific purpose.
JUnit Platform
JUnit Platform is the foundational component for testing frameworks on the Java Virtual Machine (JVM). It provides a standard interface for test discovery and execution, supporting various build tools, IDEs, and test frameworks. It includes the TestEngine API, which allows for creating custom test engines and integrating third-party testing libraries. This architecture enables seamless execution and management of tests across different testing frameworks.
JUnit Jupiter
JUnit Jupiter is the new extension of JUnit. It consists of new annotations and libraries of JUnit 5 to use in the test classes by enhancing capabilities beyond those in JUnit 4. To be more clear, JUnit Jupiter consists of two parts: Jupiter API and Jupiter Engine.
- Jupiter API: It provides a new set of annotations and assertions.
- Jupiter Engine: It helps execute tests written with the Jupiter API.
Junit Vintage
JUnit Vintage provides a Test Engine to run JUnit 4 and JUnit 3 tests, allowing users to run legacy and new tests written with JUnit 5. JUnit Vintage is a supporting library within the JUnit 5 ecosystem to ensure a smooth transition from earlier versions of JUnit.
Follow the video below to learn more about JUnit architecture and how it is better than JUnit 4.
Subscribe to the LambdaTest YouTube Channel to stay updated on video tutorials related to Selenium Java, JUnit testing, and more.
Let’s learn how to setup JUnit further, including the necessary libraries and components for effective use.
How To Install and Setup JUnit?
Here, you will get a step-by-step guide on downloading and setting up JUnit on your machine so you can use it effectively. To do so, let’s start with the pre-requisites:
Installing Java
Installing the Java Development Kit (JDK) is essential to setup JUnit for automation testing. This JDK enables you to code and run Java programs. It is recommended that you install the latest version.
Below are the steps to guide you on installing Java on Windows.
- Go to the Java SE (Standard Edition) page and click JDK Download.
- Accept the license agreement to download the JDK executable file.
- Double-click on the installer executable to begin the installation process. After installation, the JDK will be located in Program Files > Java > JDK-22 (or a similar directory, depending on your installed version).
- Upon installation of Java, you must append the installation location to the environment variables PATH and CLASSPATH.
- Search for Environment Variables in your system and select Edit the system environment variables.
- Click the Advanced tab in the System Properties window, then click the Environment Variables… button.
- Under System Variables, click New.
- Under the Variable name, enter JAVA_HOME, and for the Variable value, enter the path where your JDK is stored. Click the OK button to save the entries.
- The last step is to verify if the installation of Java on the machine was successful. To do so, run the command
java version
to verify it.
You have successfully setup Java on your machine by following the steps above. Next, let’s proceed to setup the JUnit environment variable.
Setting JUnit Environment Variable
Here, you will learn two key aspects: installing JUnit and setting up its environment variables.
Follow the steps below to complete the setup of JUnit on your machine, including downloading and installing JUnit 5.
- Go to the JUnit 5 releases page on Maven Central or the JUnit official website.
- Download the latest version of JUnit 5. You’ll typically need the following JAR files:
- junit-jupiter-api
- junit-jupiter-engine
- junit-platform-launcher
- Click on junit.jar to redirect to the Maven Central repository, where you can download the JUnit jar file.
- In the Maven Central Repository, click on the latest version of the JUnit framework (recommended latest).
- Download the Hamcrest (v2.2) JAR file from the Maven repository. The Hamcrest core jar file is now obsolete. Instead, a single Hamcrest jar file has to be downloaded so that you can execute the JUnit tests in Eclipse, IntelliJ, and on the command line.
You can add JUnit 5 to your project using a build tool like Maven or Gradle, which will handle your dependencies.
This will open a page where you can download the JUnit JAR file and view options to download the dependencies for inclusion in pom.xml. Since we are manually downloading the JUnit JAR, you must include this JAR file in your pom.xml.
Alternatively, you can download the JUnit JAR file directly from the Maven repository using this JAR file and save the JUnit JAR file in a designated location, such as C:\JUnit.
So far, we have downloaded two jar files (JUnit and Hamcrest) on our system. The files are placed in the C:\JUnit folder directory.
Now that you have completed the setup for JUnit along with the required libraries, the next step is to configure the necessary environment variables for accessing the JUnit framework.
To setup the JUnit environment variable on your machine, follow the same steps you used for setting up the Java environment variable.
Below are the steps for setting up JUnit environment variables.
- Search for Environment Variables on Windows.
- In the System Properties window, click on Environment Variables…
- Under the System Variables section, click New.
- Enter JUNIT_HOME for the Variable name and the path where JUnit is stored for the Variable value.
- Click OK to save the new environment variable.
Great, you have completed the setup of JUnit on your machine. Next, learn to configure and use JUnit in an IDE like IntelliJ.
How To Use JUnit With IntelliJ IDEA?
IntelliJ IDEA is a popular cross-platform Integrated Development Environment (IDE) developed by JetBrains. It supports Java, Kotlin, Scala, Groovy, and various other languages through plugins, including PHP, Python, Ruby, and JavaScript. IntelliJ IDEA is suitable for various development needs and is available in an Apache-licensed Community Edition and a proprietary Commercial Edition.
Setting IntelliJ IDEA Environment
Once you’ve completed the setup of JUnit on your machine, you can leverage IntelliJ IDEA to write and manage more complex test code. This IDE simplifies using the JUnit framework, allowing you to write, execute, and manage your tests efficiently.
To get started, follow these steps for the setup of JUnit in IntelliJ:
- Create a new Maven project in IntelliJ and select the project SDK.
- Name your project JUnit Demo.
- If multiple Java SDK versions are installed on your system, you can choose the desired Java SDK for your project.
- Right-click on your project and select Open Module Settings.
- Under Project Settings, click on Project.
- Select the Java SDK version “22” in the Project SDK dropdown.
- Click OK to apply the changes.
- Once the project structure is ready, you can create a new package in \src\test\java.
- You can name the package com.test.junitdemo.
- Right-click on the newly created package (e.g., com.test.junitdemo) and select New > Java Class to create a new Java class.
Alternatively, If you’re using Maven or Gradle, add JUnit dependencies to your pom.xml or build.gradle file respectively.
For Maven:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<dependencies> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <version>5.8.2</version> <!-- Use the latest version --> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-engine</artifactId> <version>5.8.2</version> <!-- Use the latest version --> <scope>test</scope> </dependency> </dependencies> |
For Gradle:
1 2 3 4 |
dependencies { testImplementation 'org.junit.jupiter:junit-jupiter-api:5.8.2' // Use the latest version testRuntimeOnly 'org.junit.jupiter:junit-jupiter-engine:5.8.2' // Use the latest version } |
Note: If not using a build tool, download the JUnit JAR files and add them to your project’s classpath.
Creating Your First JUnit Test
After completing the setup of JUnit, it is time to create your first test. Writing a basic code will help you understand how JUnit works and validate that your setup is correct.
The below class has a method that converts Fahrenheit to Celsius.
Now, let us write the simple JUnit test class to test the conversion() in Class 1.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
package com.lambdatest.junit; import org.junit.Test; import static junit.framework.Assert.assertEquals; public class JunitTestClass1 { @Test public void testconversion(){ //Given Class1 classunderTest=new Class1(); //When double temparature=80.0d; String unit=""; double result = classunderTest.conversion(temparature, unit); //Then //assertions assertEquals(170.00d,result,0.0); } } |
The JUnit annotation @Test tells JUnit that the public void method it is attached to can be run as a test case.
We split the JUnit test class into three parts: Given, When, and Then.
- Given: you can create and initialize a new class instance under test.
- When: you can setup the variables that need to be passed when calling the method under test. If the method returns a value, it must be captured in a variable for assertion.
- Then: you can assert that the actual value matches the expected value using the assertEquals(expected, actual, delta) method.
- expected is the value specified for comparison.
- actual is the value returned by the method being tested.
- delta is the margin of error for the comparison for validating floating-point values.
Here:
To learn more about JUnit assertion, watch the video below and get detailed insights.
Setting JUnit in IntelliJ IDEA
There are two ways to setup JUnit on IntelliJ IDEA:
- Adding local dependencies (to add JUnit, Hamcrest, and other relevant dependencies)
- Installing JUnit jar in IntelliJ as External Jar
- Global – Available for many projects
- Module – Available for a specific module
- Project – Available for all modules within a project
- Right-click on the project or go to File > Project Structure in the menu and select Open Module Settings.
- In the Project Settings window, select Libraries. Click the + button and choose Java.
- Navigate to the path containing the JUnit and Hamcrest JAR files. Select these files and click OK.
- The JAR files will be added as External Libraries to the project.
- To verify the successful addition of External Libraries, double-click on any JUnit import statement (e.g., import org.junit.AfterClass;). You will see that it refers to the JUnit JAR included as an External Library in the project.
- Right-click on the project and select Run ‘All Tests.’
- You can also run JUnit tests by right-clicking a particular test (e.g., JUnitDemo) and clicking Run ‘TestName’ (e.g., Run JUnitDemoClass).
- Class level: To achieve parallel execution at the class level, you must set the properties below in the junit-platform.properties file.
- Method Level: To run specific test methods in parallel within the same class, apply the @Execution annotation at the method level.
- Open the browser and navigate to LambdaTest eCommerce Playground.
- Maximize the browser window.
- Locate the search input field and enter “iPhone.”
- Click on the search button.
- Verify that the page title is “Search iphone.”
- Create an account on the LambdaTest platform.
- Get your username and access key from the LambdaTest Profile > Account Settings > Password & Security tab. These details are required for you to initiate your test on the LambdaTest platform.
- You also need to get the capabilities. These capabilities allow you to setup your test details, such as the platform you want to run your test, the browser and its version, and the name of your tests. LambdaTest Capabilities Generator is core to the process and helps bridge the gap between your local test script and executing it on a cloud platform.
- Update the desired capabilities in the above code and run the test.
- Right-click the class you want to test in the Package Explorer area on the left side of the Eclipse window.
- Click New → JUnit Test Case. This will open a dialog box to help you create your test case.
You can specify the paths to local dependencies (e.g., JUnit and Hamcrest) in the pom.xml file. If you have used pom.xml in Eclipse, you can use the same file in IntelliJ IDEA without any modifications.
Here is an example snippet from pom.xml showing how to include local dependencies:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<dependencies> [...] <dependency> <groupId>hamcrest</groupId> <artifactId>hamcrest</artifactId> <version>2.2</version> <scope>system</scope> <systemPath>C:\JUnit\hamcrest-2.2.jar</systemPath> </dependency> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.1</version> <scope>system</scope> <systemPath>C:\JUnit\junit.jar</systemPath> </dependency> [...] </dependencies> |
Double-click on any JUnit import statement to verify that the local dependencies are correctly included (e.g., import org.junit.AfterClass;). This will confirm if the local dependencies are fulfilled as specified in the pom.xml.
If you prefer not to use pom.xml, add local dependencies through the IntelliJ IDEA GUI. Dependencies are libraries on which the module depends, such as JUnit and Hamcrest.
Dependencies can be added at three levels in IntelliJ IDEA:
To install JUnit and Hamcrest JAR files as external libraries at the ‘Project Level’:
With the above steps, you have completed the setup of JUnit in IntelliJ. Now, let’s learn how to execute your JUnit tests within the IntelliJ platform.
Executing JUnit Tests in IntelliJ IDEA
To run JUnit tests in IntelliJ IDEA, follow the below steps:
The execution snapshot below shows that the test was successfully executed.
JUnit 5 supports parallel execution of tests, which speeds up the execution of the test suite when there are a large number of tests. There are two ways to achieve parallel execution in JUnit 5: at the class and method levels. Below, you can see how to configure parallel execution for both approaches.
1 2 3 |
<properties> <junit.jupiter.execution.parallel.enabled>true</junit.jupiter.execution.parallel.enabled> </properties> |
1 2 3 4 5 6 7 8 9 10 |
import org.junit.jupiter.api.Test; import org.junit.jupiter.api.parallel.Execution; import org.junit.jupiter.api.parallel.ExecutionMode; class ParallelMethodTest { @Test @Execution(ExecutionMode.CONCURRENT) void test1() { // test logic } } |
However, with parallel execution, managing test environments can become challenging. You can use a cloud-based testing platform like LambdaTest to overcome such a challenge.
LambdaTest is an AI-powered test execution platform that lets you run manual and automated tests using one or more testing frameworks in parallel over 3000+ real devices, browsers, and OS combinations.
Let us execute a small parallel test to ensure the search functionality works correctly when searching for an iPhone.
Test Scenario:
|
Expected Result:
The page title should be “Search iphone”, indicating that the search functionality works as expected. This test serves as a redundant check to ensure consistency.
Below is the code demonstrating how to implement the LambdaTest capabilities and your username and access key.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 |
package com.test.junitdemo; import org.junit.After; import org.junit.Assert; import org.junit.Before; import org.junit.Test; import org.junit.jupiter.api.parallel.Execution; import org.openqa.selenium.By; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.remote.RemoteWebDriver; import java.net.MalformedURLException; import java.net.URL; import java.util.HashMap; import static org.junit.jupiter.api.parallel.ExecutionMode.CONCURRENT; @Execution(CONCURRENT) public class JUnitDemoClass { public String username = "username"; public String accesskey = "access_key"; public static RemoteWebDriver driver; public String gridURL = "@hub.lambdatest.com/wd/hub"; boolean status = false; static String URL = "https://ecommerce-playground.lambdatest.io/"; @Before public void setup() throws MalformedURLException { String hub = "@hub.lambdatest.com/wd/hub"; ChromeOptions browserOptions = new ChromeOptions(); browserOptions.setPlatformName("Windows 11"); browserOptions.setBrowserVersion("126.0"); HashMap<String, Object> ltOptions = new HashMap<String, Object>(); ltOptions.put("username", "username"); ltOptions.put("accessKey", "acceeskey"); ltOptions.put("project", "Junit Test"); ltOptions.put("w3c", true); ltOptions.put("plugin", "java-testNG"); browserOptions.setCapability("LT:Options", ltOptions); try { driver = new RemoteWebDriver(new URL("https://" + username + ":" + accesskey + gridURL), browserOptions); } catch (MalformedURLException e) { System.out.println("Invalid grid URL"); } catch (Exception e) { System.out.println(e.getMessage()); } } @Test public void test_() throws InterruptedException { System.out.println(Thread.currentThread().getName()); driver.navigate().to(URL); driver.manage().window().maximize(); try { driver.findElement(By.xpath("(//input[@placeholder='Search For Products'])[1]")).sendKeys("iPhone"); driver.findElement(By.xpath("//button[normalize-space()='Search']")).click(); String actualTitle = driver.getTitle(); Assert.assertEquals(actualTitle, "Search IPhone"); if (actualTitle.equals("Search-iphone")) { System.out.println("Demonstration of running JUnit tests is complete"); } } catch (Exception e) { System.out.println(e.getMessage()); } } @Test public void first() throws Exception{ System.out.println("FirstParallelUnitTest first() start => " + Thread.currentThread().getName()); Thread.sleep(500); System.out.println("FirstParallelUnitTest first() end => " + Thread.currentThread().getName()); } @Test public void second() throws Exception{ System.out.println("FirstParallelUnitTest second() start => " + Thread.currentThread().getName()); Thread.sleep(500); System.out.println("FirstParallelUnitTest second() end => " + Thread.currentThread().getName()); } @Test public void test_1() throws InterruptedException { System.out.println(Thread.currentThread().getName()); driver.navigate().to(URL); driver.manage().window().maximize(); try { driver.findElement(By.xpath("(//input[@placeholder='Search For Products'])[1]")).sendKeys("iPhone"); driver.findElement(By.xpath("//button[normalize-space()='Search']")).click(); String actualTitle = driver.getTitle(); Assert.assertEquals(actualTitle, "Search IPhone"); if (actualTitle.equals("Search-iphone")) { System.out.println("Demonstration of running JUnit tests is complete"); } } catch (Exception e) { System.out.println(e.getMessage()); } } @After public void tearDown() { driver.quit(); } } |
To use LambdaTest to perform parallel testing, follow the steps below.
Result:
You can view the execution results on the LambdaTest platform. To do this, navigate to the Automation menu on the left side and click on Web Automation.
Note: JUnit 5 uses a dynamic number thread count by default based on the availability of processors. You can limit the maximum number of threads by setting the following:
1 2 |
junit.jupiter.execution.parallel.config.strategy=fixed junit.jupiter.execution.parallel.config.fixed.parallelism=4 |
Conclusion
JUnit is one of the popular open-source test frameworks used for unit testing with Java. When using JUnit, you can add the dependencies dynamically using Maven or add the respective dependencies as ‘local dependencies’ (or External Libraries).
JUnit can be used with a local Selenium Grid and a cloud-based Selenium Grid like LambdaTest. JUnit helps to write unit tests to find and fix bugs in the early stage of development. It also helps accelerate the development and deliver error-free software.
Frequently Asked Questions (FAQs)
How do I setup a JUnit test?
You must create a separate .java file to setup the JUnit test. It should be in the same project that will test one of your existing classes.
What is a JUnit Test?
A JUnit Test is a Java test that uses the JUnit Framework to ensure the specific code units are working as expected.
What is the need for JUnit testing?
JUnit testing is needed to find the bugs in the early stage of development, which increases code readability. It also makes the source code more readable and error-free.
What are the annotations present on JUnit 5?
@BeforeAll: runs before all test methods in the current class.
@AfterAll: runs after all test methods in the current class.
@BeforeEach: runs before each test method.
@AfterEach: runs after each test method.
@Disable: disables a test class or a method.
@Tag: tags the test methods such as Smoke, Regression, Critical, etc.
@Nested: annotated class is a nested, non-static test class.
@DisplayName: declares a custom display name for a test class or method.
@ExtendWith: it is used to register custom extensions.
@TestFactory: declares that the method is a test factory for dynamic testing.
Got Questions? Drop them on LambdaTest Community. Visit now