How to Automate Filling In Web Forms With Python
Paulo Oliveira
Posted On: July 11, 2024
794782 Views
11 Min Read
Web forms are key components for data entry and interaction across various business processes. So, it’s important to ensure the functionality of these forms across the website. However, testing forms manually is both time-consuming and prone to error. This is where automated form filling in your test process can significantly enhance productivity and accuracy, especially in data-driven tasks.
Programming languages such as Python, with its simple and readable syntax, offer a highly effective solution for such automation. This tutorial will look at how to automate filling in web forms with Python.
TABLE OF CONTENTS
Why Use Automated Form Filling?
Automated form filling in automation testing is crucial for various use cases, including login, registration, eCommerce checkout, and dynamic forms. It ensures accurate data entry, proper validation, and smooth submissions, speeding up the testing process and providing consistent, reliable results.
Automation allows extensive test coverage, simulates multiple users for functional testing, and ensures new changes don’t break existing functionality. For this, different automation testing tools like Selenium can help implement automated form fillings with Python.
If you want to learn more about Selenium, check out this tutorial: what is Selenium.
Types of Web Forms and Input Fields
Automated form filling involves managing different kinds of forms and input fields.
Types of Web Forms:
- Dynamic Forms: Forms that change based on prior inputs require scripts capable of handling conditional logic. Automation tools can adjust to these changes, ensuring the form is filled out correctly based on its current state.
- Multi-Step Forms: These forms extend over several pages or steps. Automation scripts need to navigate through each step, ensuring data is entered accurately and transitions between steps are managed smoothly.
Subscribe to the LambdaTest YouTube Channel and stay updated with the latest video tutorials on Selenium testing and more!
Types of Input Fields:
- Input Texts and Text Areas: These are the most known and frequent input types, used for short and long text entries. Automation scripts can address these by sending the required text directly into the fields.
- Dropdown Lists: Dropdowns require scripts to choose options from a given list. This is done by targeting the dropdown element and selecting the appropriate choice based on its value or displayed text. You can learn more about dropdowns from this blog on handling dynamic dropdowns in Selenium.
- Radio Buttons and Checkboxes: These elements allow users to make single or multiple selections. When handling radio buttons in Selenium, automation scripts can interact with these by clicking on the necessary options, ensuring the correct selections are made.
- Date Pickers: Date pickers often include specialized widgets for picking dates. When handling date pickers in Selenium, automating these fields may involve interacting with the date picker interface or directly inputting the date in the required format.
- File Upload Fields: Some forms require file uploads. When uploading files in Selenium, automated test scripts simulate the file selection dialog and provide the file path, ensuring the correct file is uploaded.
- CAPTCHA and reCAPTCHA: Although it was designed to block automatic submissions, there are solutions for handling these in testing environments, such as using test keys provided by CAPTCHA services. Refer to this blog to learn how to handle CAPTCHA in Selenium.
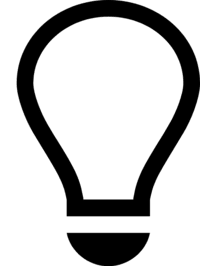
Test Your Forms and Input Fields Across 3000+ Environments. Try LambdaTest Today!
Demo: How to Automate Filling In Web Forms With Python?
Before demonstrating how to automate filling in web forms with Python, we need to proceed with the following steps:
- Install Python according to your operating system.
- Install Selenium using the pip install selenium.
- Install VS Code or free to use your preferred IDE.
Test Scenario:
- Open the Register Account form in the LambdaTest eCommerce Playground.
- Fill in the details.
- Fill in the password.
- Subscribe to the Newsletter.
- Accept the terms and conditions.
- Click the Continue button.
- Check that a success message is shown to the user.
Implementation:
Here is our test script to automate web form filling using Python with Selenium:
Code Walkthrough:
Here is the snippet from the main.py file that automates the web form-filling process on the Register Account form of the LambdaTest eCommerce Playground website.
Import the Selenium WebDriver, which is required to interact with the browser. Then, you have to instantiate the driver in a variable called browser. In this case, we are instantiating the ChromeDriver, given that we will use it in the Chrome browser.
Finally, you need to use the browser instance to access the desired web page, using the get() function and inform the website URL that it should open. If you want a better view, you can maximize your browser window using the browser.maximize_window() method.
Use element locators like ID to find and fill the First Name, Last Name, Email, and Telephone fields:
Locate Password fields by ID and input values. You can use XPath when IDs are unavailable, such as subscribing to a Newsletter:
Locate and click the Submit button using the click() method. We have the assert keyword that compares two values. The first value will be the browser title value. We can get the browser title value using the property title of the browser instance. The second value will be the expected value, which should be Your Account Has Been Created!
Use the browser.quit() method to close the browser window.
Now, you know how to automate filling in web forms with Python. In this section, we have run the test on the Chrome browser. However, to test your web form fillings across various web browsers and their browser versions, you can use cloud-based testing platforms like LambdaTest.
How to Automate Filling In Web Forms on the Cloud?
In this section, we will look at how to test the web form fillings using online Selenium Grid like LambdaTest. Here, we will use the same test scenario of the Register Account form.
LambdaTest is an AI-powered test execution platform that helps developers and testers ship code faster. It provides 3000+ browsers and operating systems to perform Selenium Python testing at scale.
Looking to ace your next tech interview? Check out our comprehensive guide on Operating System Interview Questions.
Implementation:
Following are some changes you need to make in your test scripts to implement automated form filling on the LambdaTest platform. Here is our final test script:
Code Walkthrough:
Remove or comment out the line that instantiates the WebDriver:
1 |
#browser = webdriver.Chrome() |
Configure your username, accessToken, and gridUrl. You can get them from the LambdaTest Dashboard by navigating to Settings > Account Settings > Password & Security.
1 2 3 |
username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Token" gridUrl = "hub.lambdatest.com/wd/hub" |
The main difference between running the tests locally or on LambdaTest is to use the capabilities to configure the environment where the test will run. You can automatically generate these from the Automation Capabilities Generator.
1 2 3 4 5 6 7 8 9 10 |
lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } |
Instantiate a ChromeOption object and set its capabilities with the above-configured lt_options variable:
1 2 3 |
web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) |
Set the URL where the test will run, with a default format, and the test script that will instantiate the driver in a variable called browser.
1 2 3 4 5 |
url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, desired_capabilities=lt_options ) |
Run your test in LambdaTest by just passing the below command:
1 |
python main-cloudgrid.py |
Test Execution:
Visit the LambdaTest Web Automation Dashboard, and you can see the test execution results:
On a side note, you can further enhance your Selenium automation testing by leveraging AI testing agents like KaneAI.
KaneAI is a smart AI test assistant for high-speed quality engineering teams. With its unique AI-driven features for test authoring, management, and debugging, KaneAI allows teams to create and evolve complex test cases using natural language.
Best Practices for Effective Web Form Automation
To achieve successful web form automation, it’s essential to follow certain best practices. These recommendations ensure your automation scripts are robust, reliable, and efficient:
- Use Unique Selectors: Interact with elements using unique selectors, such as IDs or unique class names. This reduces the chances of your script failing due to changes in the web page structure.
- Strategic Use of Waits: Implement implicit and explicit waits to manage the asynchronous loading of web elements. This prevents your script from interacting with elements that need to be fully loaded. For Python web automation, LambdaTest offers a SmartWait that eliminates the use of multiple waits in test scripts, thereby reducing the test execution time.
- Implement Design Patterns: Organize your automated test scripts by implementing design patterns like the Page Object Model (POM) in Selenium Python. These patterns enhance maintainability by separating the page representation from the test scripts, making your code more modular and easier to manage.
- Perform Cross Browser Testing: Ensure your automation scripts test forms across different browsers and devices to ensure functionality for a broader audience.
- Leverage Data-Driven Testing: Use data-driven testing to handle multiple test cases with input data sets. This approach increases test coverage and simplifies the management of extensive test data.
Discover the top Python frameworks to watch out for in 2025. Stay updated on the tools shaping the future of development.
Conclusion
In this blog on how to automate filling in web forms with Python, we explored setting up Selenium Python for automating web form filling. We started with the basics: loading a web page in Chrome using Python. Next, we delved into automating web form filling, covering various fields and locator strategies. Finally, we discussed testing web forms using the LambdaTest platform.
Frequently Asked Questions (FAQs)
How to fill a form on a website using Python?
To fill out a form on a website using Python, you can use frameworks like Selenium. Selenium automates browsers, allowing you to programmatically enter data into form fields, simulate button clicks, and navigate the website as needed.
How to autofill a form using Python?
Selenium is a popular choice for auto-filling a form using Python. It lets you control a web browser, locate form fields using XPath or CSS Selectors, and automatically fill them with predefined data, which is ideal for testing or repetitive form submissions.
How to automate filling out a web form?
Automating the process of filling out web forms can be efficiently achieved with frameworks such as Selenium. By leveraging its capabilities, you can programmatically enter values into text boxes, select options from dropdowns, and handle various form controls like checkboxes and radio buttons to mimic human interaction.
Got Questions? Drop them on LambdaTest Community. Visit now