ExpectedConditions In Selenium: Types And Examples
Faisal Khatri
Posted On: August 13, 2024
365033 Views
21 Min Read
ExpectedConditions in Selenium allow you to wait for specific conditions to be met before proceeding with interactions with the WebElements. This is common when the WebElement is not yet present or is visible but not clickable or text attributes are not being updated in time.
Instead of relying on fixed wait times (Implicit Wait), ExpectedConditions in Selenium enables you to wait till the time WebElements become visible or clickable, thus ensuring your tests run smoothly even in dynamic environments.
What Are ExpectedConditions in Selenium?
ExpectedConditions are used to perform Explicit Waits on specific conditions, ensuring that Selenium WebDriver waits for those conditions to be met before proceeding with further actions. This clarification helps in understanding how ExpectedConditions provide the necessary wait time between actions, such as locating a WebElement or performing other operations.
There are multiple benefits of using ExpectedConditions in Selenium test automation, some of which are listed below.
- Reduces Test Flakiness: It ensures that the specific conditions are met before proceeding, making test scripts more reliable and reducing the chances of false failures.
- Reduces Test Execution Time: By waiting only for specific conditions on certain WebElements, ExpectedConditions optimize the wait time, improving overall test execution efficiency.
- Allows Better Implementation of Waits: Selenium WebDriverWait and the methods provided by the ExpectedConditions class enable more precise and effective waits tailored to the specific needs of your test, enhancing overall test reliability.
- Enhances Readability: It clearly specifies the conditions that need to be met for the code to proceed to the next step. This enhances the code’s readability and maintainability.
Types of ExpectedConditions In Selenium
ExpectedConditions in Selenium offer commonly used conditions for automating test scenarios. It allows you to implement Explicit Waits directly in your test code. Follow this blog on Selenium Waits to learn more about Implicit, Explicit, and Fluent Waits in detail.
Below are the types of ExpectedConditions in Selenium.
ExpectedCondition<WebElement>
The ExpectedCondition <WebElement> uses the locator of a WebElement as a parameter. The Explicit Wait continues until the specified condition is met or the wait duration expires. If the condition is satisfied, it returns the WebElement, a list of WebElements, or other relevant information based on the specific ExpectedCondition.
For example, the numberOfElementsToBeLessThan method returns true or false depending on whether the number of WebElements located by the given locator is less than the expected number. The elementToBeClickable method returns a WebElement if it is determined to be clickable.
ExpectedCondition<WebDriver>
This category of ExpectedCondition returns a WebDriver instance after the required operation is successfully performed.
For example, the frameToBeAvailableAndSwitchToIt method switches the WebDriver to a specified frame located by the given frame locator (ID or Name). If the frame is not available, an exception is raised.
ExpectedCondition<Boolean>
The ExpectedCondition of type <Boolean> takes a String parameter, applying the wait to the condition specified by the parameter. It returns true if the condition is met and false if it is not.
For example, the textToBePresentInElementLocated method returns true when the WebElement located by the given locator contains the specified text.
ExpectedCondition<Alert>
This category of ExpectedCondition returns an alert if an Alert window is present on the page. Once the alert is detected, the WebDriver switches to it, allowing operations like accept(), dismiss(), sendKeys(), and getText(). If the Alert window is not available within the specified wait duration, the method returns null.
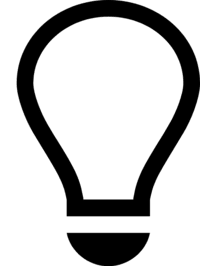
Run your tests across 3000+ browsers and OS combinations. Try LambdaTest Today!
Below are some of the commonly used methods provided by ExpectedConditions in Selenium for automation testing.
Commonly Used Methods With ExpectedConditions
Now that we have covered the various types of ExpectedConditions in Selenium, in this section, you can have a look at some of the widely used methods of ExpectedConditions for realizing Explicit Waits. We will be executing the tests on the LambdaTest platform.
LambdaTest is an AI-powered test execution platform that lets you conduct manual and automated tests at scale with over 3000+ browsers and OS combinations.
This platform provides ready-to-use test infrastructure with a wide range of automation testing frameworks to select and use simultaneously. You can get started with this platform using the simple steps below.
- Create an account on LambdaTest.
- Get your username and access key from the Profile > Account Settings > Password & Security tab.
- Use the LambdaTest Capabilities Generator to configure your test setup. You can specify where you want to run the test and select the platform, automation frameworks, browser, browser version, and other capabilities. These capabilities must then be added to your local test script.
To implement the capabilities in your test script, create a file called BaseTest.java. This file will contain the basic LambdaTest configuration, including setup and teardown methods for opening and closing the browser.
Before creating and adding methods to BaseTest.java, it’s important to put the necessary libraries in one place. To do so, you can add the dependencies to pom.xml.
The pom.xml configuration file helps maintain the project dependencies. Shown below is the complete implementation of pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" | |
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> | |
<modelVersion>4.0.0</modelVersion> | |
<groupId>io.github.mfaisalkhatri</groupId> | |
<artifactId>selenium-lambdatest-demo</artifactId> | |
<version>1.0-SNAPSHOT</version> | |
<packaging>jar</packaging> | |
<name>selenium-lambdatest-demo</name> | |
<url>http://maven.apache.org</url> | |
<properties> | |
<selenium-java.version>4.23.0</selenium-java.version> | |
<testng.version>7.10.2</testng.version> | |
<maven.compiler.version>3.13.0</maven.compiler.version> | |
<surefire-version>3.3.1</surefire-version> | |
<java.release.version>17</java.release.version> | |
<maven.source.encoding>UTF-8</maven.source.encoding> | |
<suite-xml>test-suites/testng.xml</suite-xml> | |
<argLine>-Dfile.encoding=UTF-8 -Xdebug -Xnoagent</argLine> | |
</properties> | |
<dependencies> | |
<dependency> | |
<groupId>org.seleniumhq.selenium</groupId> | |
<artifactId>selenium-java</artifactId> | |
<version>${selenium-java.version}</version> | |
</dependency> | |
<dependency> | |
<groupId>org.testng</groupId> | |
<artifactId>testng</artifactId> | |
<version>${testng.version}</version> | |
<scope>test</scope> | |
</dependency> | |
</dependencies> | |
<build> | |
<plugins> | |
<plugin> | |
<groupId>org.apache.maven.plugins</groupId> | |
<artifactId>maven-compiler-plugin</artifactId> | |
<version>${maven.compiler.version}</version> | |
<configuration> | |
<release>${java.release.version}</release> | |
<encoding>${maven.source.encoding}</encoding> | |
<forceJavacCompilerUse>true</forceJavacCompilerUse> | |
</configuration> | |
</plugin> | |
<plugin> | |
<groupId>org.apache.maven.plugins</groupId> | |
<artifactId>maven-surefire-plugin</artifactId> | |
<version>${surefire-version}</version> | |
<executions> | |
<execution> | |
<goals> | |
<goal>test</goal> | |
</goals> | |
</execution> | |
</executions> | |
<configuration> | |
<useSystemClassLoader>false</useSystemClassLoader> | |
<properties> | |
<property> | |
<name>usedefaultlisteners</name> | |
<value>false</value> | |
</property> | |
</properties> | |
<suiteXmlFiles> | |
<suiteXmlFile>${suite-xml}</suiteXmlFile> | |
</suiteXmlFiles> | |
<argLine>${argLine}</argLine> | |
</configuration> | |
</plugin> | |
</plugins> | |
</build> | |
</project> |
In the BaseTest class, located in the package expectedconditionsdemo, you will configure the settings required for running tests on the LambdaTest cloud grid.
public class BaseTest { | |
protected RemoteWebDriver driver; | |
@BeforeClass | |
public void setup() { | |
String USERNAME = System.getenv("LT_USERNAME") == null ? "LT_USERNAME" : System.getenv("LT_USERNAME"); | |
String ACCESS_KEY = System.getenv("LT_ACCESS_KEY") == null ? "LT_ACCESS_KEY" : System.getenv("LT_ACCESS_KEY"); | |
String GRID_URL = "@hub.lambdatest.com/wd/hub"; | |
try { | |
this.driver = new RemoteWebDriver(new URL("http://" + USERNAME + ":" + ACCESS_KEY + GRID_URL), getChromeOptions()); | |
} catch (final MalformedURLException e) { | |
System.out.println("Could not start the remote session on LambdaTest cloud grid"); | |
} | |
this.driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(20)); | |
} | |
public ChromeOptions getChromeOptions() { | |
final var browserOptions = new ChromeOptions(); | |
browserOptions.setPlatformName("Windows 10"); | |
browserOptions.setBrowserVersion("126.0"); | |
final HashMap<String, Object> ltOptions = new HashMap<String, Object>(); | |
ltOptions.put("project", "Expected Conditions Blog"); | |
ltOptions.put("build", "LambdaTest"); | |
ltOptions.put("name", "Expected Conditions Demo"); | |
ltOptions.put("visual", true); | |
ltOptions.put("network", true); | |
ltOptions.put("console", true); | |
ltOptions.put("w3c", true); | |
ltOptions.put("plugin", "java-testNG"); | |
browserOptions.setCapability("LT:Options", ltOptions); | |
return browserOptions; | |
} | |
@AfterClass | |
public void tearDown() { | |
this.driver.quit(); | |
} | |
} |
In the above code, the setup() method in the BaseTest class sets up the Selenium RemoteWebDriver instance, using the getChromeOptions() method to pass the required capabilities such as platform name, browser version, build, and test name.
The tearDown() method, called after tests are executed, will gracefully close the RemoteWebDriver session. The setup() and tearDown() methods should be implemented under the @BeforeClass and @AfterClass annotations, respectively, in the BaseTest class.
Below are examples that demonstrate a few of the widely used methods with ExpectedConditions in Selenium.
elementToBeSelected
It is an overloaded method that belongs to the ExpectedCondition <Boolean> category.
ExpectedCondition <Boolean>elementToBeSelected(finalBy locator)
This ExpectedCondition waits until the WebElement specified by the locator is selected. It returns true if the element is selected and false otherwise.
Syntax:
ExpectedCondition <Boolean>elementToBeSelected(final WebElement element)
This ExpectedCondition takes a WebElement as a parameter and waits until the element is selected. It internally calls elementSelectionStateToBe() with the WebElement and the Boolean value true.
Syntax:
To better understand how the elementToBeSelected() method works with ExpectedConditions in Selenium, let’s consider a test scenario.
Test Scenario:
|
Code Implementation:
A new test method testElementToBeSelected() is created in the existing ExpectedConditionsDemoTest class for implementing the test scenario.
Code Walkthrough:
Below is the code walkthrough for using the testElementToBeSelected() method with ExpectedConditions in Selenium:
- Locate the “Check All” button: Use the ID locator to find the “Check All” button and perform a click event on it.
- Wait for Checkboxes to be Selected: Use the testElementToBeSelected() method from the ExpectedConditions class to wait until “Checkbox 1” and “Checkbox 2” are selected.
- Verify Selection: The testElementToBeSelected() method returns a boolean value (true or false) based on the element’s selection. Wrap this statement in the assertTrue() method for inline verification to ensure that the checkboxes are selected.
Test Execution:
The following screenshots show the successful execution of the tests running on IntelliJ IDE.
The test details are displayed on the LambdaTest dashboard as well.
elementToBeClickable
The elementToBeClickable() method of ExpectedConditions in Selenium is an overloaded method that belongs to the ExpectedCondition <WebElement> category. This method waits till the WebElement is visible and enabled so that the click operation can be performed on it.
ExpectedCondition <WebElement>elementToBeClickable(By locator)
Selenium WebDriver waits until the element, identified by the specified locator, is visible and enabled before allowing a click action.
Syntax:
ExpectedCondition<WebElement<elementToBeClickable(WebElement element)
Selenium WebDriver waits until the WebElement is passed as a visible parameter and enabled before clicking it.
Syntax:
To better understand how the elementToBeClickable() method works with ExpectedConditions in Selenium, let’s consider a test scenario.
Test Scenario :
|
Code Implementation:
A new test method, testElementToBeClickableCondition(), is created in the existing ExpectedConditionsDemo class to implement the test scenario.
Code Walkthrough:
Below is the code walkthrough for the testElementToBeClickableCondition() method:
- Navigate to the Simple Form Demo Page: The test method will first navigate to the Simple Form Demo page.
- Locate the “Enter Message” Field: Use the ID locator strategy to find the “Enter Message” field.
- Enter Text: Use the sendKeys() method to input the text “This is a test” into the “Enter Message” field.
- Instantiate WebDriverWait: To use the ExpectedConditions class, instantiate the WebDriverWait class by passing the WebDriver instance and the desired wait duration as constructor parameters.
- Locate the “Get Checked Value” Button: Use the elementToBeClickable() method from the ExpectedConditions class to locate the “Get Checked Value” button. The WebDriver will wait up to 30 seconds for the button to become clickable.
- Click the Button: Once the button becomes clickable, perform the click action.
- Verify the Input Message: Finally, retrieve and verify the text displayed below “Your Message” to confirm that it matches the input message.
Test Execution:
The following screenshots show the successful execution of the tests running on IntelliJ IDE.
The test details are displayed on the LambdaTest dashboard as well.
visibilityOfElementLocated
The visibilityOfElementLocated() method checks if the specified element is not only present in the DOM but also visible on the page. This method takes a locator as a parameter to find the element and returns the WebElement once it is both located and visible.
Syntax:
To better understand how the visibilityOfElementLocated() method works with ExpectedConditions in Selenium, let’s consider a test scenario.
Test Scenario:
|
Code Implementation:
In the existing ExpectedConditionsDemoTest class, add a new test method, testVisibilityOfElementLocated(), to implement the test scenario.
Code Walkthrough:
Below is the code walkthrough for the testVisibilityOfElementLocated() method:
- Navigation: The script navigates to the “Drag and Drop Demo” on LambdaTest Selenium Playground.
- Element Identification: “Draggable 1” is located using the CSS Selector strategy.
- Drag-and-Drop Action: The dragAndDrop() method from the Actions class is used, with parameters for the draggable item and the drop target.
- Visibility Check: The script waits for the “Dropped Item List” to appear using the testVisibilityOfElementLocated() method from ExpectedConditions.
- Assertion: An assertion confirms that the item in the “Dropped List” matches the one dragged.
Test Execution:
The following screenshots show the successful execution of the tests running on IntelliJ IDE.
The test details are displayed on the LambdaTest dashboard as well.
textToBePresentInElementLocated
This method checks whether the specified text passed as a parameter is present in the WebElement that matches the given locator. It returns true if the text is present; otherwise, it returns false.
Syntax:
To better understand how the textToBePresentInElementLocated() method works with ExpectedConditions in Selenium, let’s consider a test scenario.
Test Scenario:
|
Code Implementation:
The testTextToBePresent() test method is added to the existing ExpectedConditionsDemoTest class to implement the test scenario.
Code Walkthrough:
Below is the code walkthrough for the textToBePresentInElementLocated() method:
- Navigate using the driver.get() method: This method will navigate to the “Dynamic Data Loading” page on the LambdaTest Selenium Playground website. It will locate the “Get Random User” button using the ID locator strategy. Click on it.
- Use textToBePresentInElementLocated(): In this method of the ExpectedConditions class, the test will wait for the dynamic data to be loaded and check if the text “First Name” is present in the data loaded.
- Verify with assertTrue(): This condition is wrapped inside the assertTrue() method. The assertTrue() method checks that the condition is true, meaning that the text is present. If the text is not present, it will return false, and the test will fail.
Test Execution:
The following screenshots show the successful execution of the tests running on IntelliJ IDE.
The test details are displayed on the LambdaTest dashboard as well.
frameToBeAvailableAndSwitchToIt
This method checks if a given frame is available to switch to in Selenium. Frames can be identified using a locator, frame index (integer), frame name (string), or WebElement. The frameToBeAvailableAndSwitchToIt() method is overloaded, allowing you to locate and check a frame using any of these options. If the frame or iFrame is present on the page, the method triggers driver.switchTo().frame, shifting the focus to that frame.
ExpectedCondition<WebDriver>frameToBeAvailableAndSwitchToIt (By locator)
This method checks if the given frame is available to switch to. The required frame is located using the web locator passed to the method. If successful, it switches to the specified frame; otherwise, it returns null.
Syntax:
ExpectedCondition<WebDriver>frameToBeAvailableAndSwitchToIt(int frameLocator)
This method checks if the given frame can be switched to using the specified frameIndex (an integer) or frameLocator. If successful, it switches to the frame.
Syntax:
ExpectedCondition<WebDriver>frameToBeAvailableAndSwitchToIt (WebElement locator)
This method locates the frame using the provided WebElement. If successful, it switches to the frame.
Syntax:
ExpectedCondition
This method locates the frame using the frame name provided as a parameter. If the frame with the specified name is found on the page, it switches to that frame; otherwise, it returns null.
Syntax:
Handling frames and windows in Selenium can be challenging. To better understand how ExpectedConditions in Selenium helps with handling frames, let’s consider a test scenario.
Test Scenario:
|
Code Implementation:
A new test method, testFrameToBeAvailableAndSwitch(), is created in the existing ExpectedConditionsDemoTest class to implement the test scenario.
Code Walkthrough:
Below is the code walkthrough for the testFrameToBeAvailableAndSwitch() method:
- Navigate: The testFrameToBeAvailableAndSwitch() method navigates to the Simple iFrame Demo page on the LambdaTest Selenium Playground website.
- Locate and switch to the iFrame: The iFrame is located and switched to using the testFrameToBeAvailableAndSwitchToIt() method from the ExpectedConditions class.
- Locate Editor: After switching, the editor inside the iFrame is located.
- Clear any existing text: The existing text in the editor is cleared using the clear() method, and the new text “switched to iframe” is entered.
- Print the message: The message “Text entered in iFrame” is printed to the console to confirm successful execution.
Test Execution:
The following screenshots show the successful execution of the tests running on IntelliJ IDE.
The test details are displayed on the LambdaTest dashboard as well.
alertIsPresent
The alertIsPresent() method, as the name suggests, checks if an Alert window is available on the page. Depending on the type of Alert (Simple Alert, Prompt Alert, or Confirmation Alert), appropriate actions should be performed. If the Alert window is present, this method internally triggers the driver.switchTo().alert() command, shifting the focus to the Alert window.
Syntax:
To better understand how the alertIsPresent() method works with ExpectedConditions in Selenium, let’s consider a test scenario.
Test Scenario:
|
Code Implementation:
A new test method, testJavaScriptAlert() is created in the existing ExpectedConditionsDemoTest class to implement the test scenario.
Code Walkthrough:
Below is the code walkthrough for the testJavaScriptAlert() method:
- Navigation: The code navigates to the JavaScript alert window on the LambdaTest Selenium Playground website.
- Element Location: The “Click Me” button is located using the CSS Selector locator strategy, and a click action is performed.
- Alert Handling:
- Wait for Alert: The alertIsPresent() method from the ExpectedConditions class is used to wait for the alert to appear. This method will wait until the alert is present and then switch to it.
- Capture and Verify Alert Text: The text of the alert is captured in the alertText variable and verified to ensure the alert appears as expected.
- Accept Alert: The accept() method is called to accept the alert, effectively clicking the OK button to close it.
Test Execution:
The following screenshots show the successful execution of the tests running on IntelliJ IDE.
The test details are displayed on the LambdaTest dashboard as well.
presenceOfElementLocated
The presenceOfElementLocated() method waits for a specified WebElement to be present in the DOM of the page. However, the presence of an element in the DOM does not necessarily mean that the element is visible. This method takes a locator as a parameter to find the element on the page and returns the WebElement once it is located.
Syntax:
titleIs
This method checks whether the title of the current page matches the expected title. It returns true if the title matches with the expected title. It returns false if the titles do not match.
Syntax:
titleContains
This method checks whether the current page title or the title of a WebElement contains a specific case-sensitive substring. It returns true if the substring is present in the title.
Syntax:
textToBePresentInElementLocated
This method checks if the given text (passed as a parameter to the method) is present in the WebElement that matches the given web locator. It returns the Boolean value true if the specified text is present in the element; otherwise, it returns false.
Syntax:
urlToBe
This method checks whether the current page URL matches (or is the same as) the URL passed as a parameter to the urlToBe() method. It returns true if the current page URL is the same as the expected URL (in the parameter); otherwise, it returns false.
Syntax:
The methods discussed above are some of the most commonly used in ExpectedConditions in Selenium. They play a vital role in ensuring that the test script waits until elements are ready and visible for interaction. ExpectedConditions in Selenium are particularly helpful when dealing with challenging elements like frames, iframes, windows, and alerts, making automation of these components easier.
Every testing scenario is unique, depending on the application being automated, so there may be instances where creating custom ExpectedConditions in Selenium is necessary.
Custom ExpectedConditions In Selenium
There are scenarios where you might want to combine multiple conditions into one. For example, a test might need to check both the visibility of an element and its clickability. This can be achieved by creating a custom ExpectedCondition in Selenium.
A custom ExpectedCondition is a class that:
- Implements the ExpectedCondition interface (e.g., ExpectedCondition
, ExpectedCondition , etc.). - Optionally, it has a constructor with parameters for the ExpectedCondition.
- Overrides the apply method using the @Override annotation in Java.
Here is a sample implementation of a custom ExpectedCondition in Selenium Java:
Let’s look at how to create custom ExpectedConditions in Selenium by automating the following test scenario:
Test Scenario:
|
Code Implementation:
A new test method testCustomExpectedCondition() is created in the existing ExpectedConditionsDemoTest class. This method will implement the test scenario for table data search using custom expected conditions.
Code Walkthrough:
Below is the code walkthrough for the testCustomExpectedCondition() method:
- Navigation: The test navigates to the Table Sorting and Searching page on the LambdaTest Selenium Playground website.
- Element Location: The search box is located using the CSS Selector locator strategy.
- Custom Expected Condition: A custom expected condition is applied to locate the table and verify a record within it. The ExpectedCondition will return true if the condition is met and false otherwise.
- First Condition: Checks if the table is displayed. If it is, the test locates the table row and then the Name column.
- If the table is not displayed, the test fails, returning false, and the condition will time out after 10 seconds.
- Second Condition: If the table is displayed, it verifies that the Name column is visible and the value in the first row of the Name column is Bennet (the searched value).
- If both conditions are met, the test passes. If the second condition fails, it returns false, marking the test as failed.
Test Execution:
The following screenshots show the successful execution of the tests running on IntelliJ IDE.
The test details are displayed on the LambdaTest dashboard as well.
To learn more about performing automation testing on the LambdaTest platform, watch the video below for detailed insights.
You can also subscribe to the LambdaTest YouTube Channel and stay ahead with the latest video tutorials on various automation testing techniques.
Conclusion
Expected Conditions are used to implement Explicit Waits in Selenium, offering significant advantages over Implicit Waits. With Implicit Waits, the wait is applied for the entire duration, even if the WebElement is located much earlier. In contrast, Explicit Waits with ExpectedConditions only pause the script until a specified condition is met, making the process more efficient.
There are four major categories of ExpectedConditions in Selenium Java: ExpectedCondition<Boolean>, ExpectedCondition<WebDriver>, ExpectedCondition<WebElement>, and ExpectedCondition<Alert>. Custom ExpectedConditions are particularly useful in Selenium automation testing when you need to combine different conditions to accomplish a specific task. These custom conditions implement the ExpectedCondition interface and override the apply method.
Explicit Waits with ExpectedConditions provide a significant advantage over Implicit Waits and should be used whenever applicable in test implementation.
Frequently Asked Questions (FAQs)
What are the expected conditions in Selenium?
Expected Conditions in Selenium are used to perform Explicit Waits. The WebDriver waits for specific conditions before it can proceed with the execution of the tests.
What are some expected conditions that can be used in Explicit Waits?
Some of the Expected Conditions that can be used in Explicit Wait are as follows:
- presenceOfElementLocated()
- visibilityOfElementLocated()
- alertIsPresent()
- elementToBeClickable()
- textToBePresentInElementLocated()
- frameToBeAvailableAndSwitchToIt()
- elementToBeSelected()
What is an explicit condition in Selenium?
An explicit condition in Selenium allows the user to wait for a specific condition to be met before continuing the test execution. WebDriverWait class can be used to implement Explicit Waits in Selenium. The ExpectedConditions class provides the different conditions that could be used for Explicit waits.
Got Questions? Drop them on LambdaTest Community. Visit now