Cypress Logs: How to Improve Custom Commands Visibility
Uma Victor
Posted On: February 12, 2025
110212 Views
14 Min Read
Cypress automatically logs the commands you use in your tests, showing you what happens during test runs. However, when you create custom commands, Cypress only tells you when those commands are used, not what happens inside them. It can make it tough to figure out what’s going wrong if something doesn’t work.
To solve this, you can add your own Cypress logs to custom commands. By doing this, you’ll see important details and actions taken at each step of your tests. This will make it much easier to troubleshoot issues and understand how your tests interact with your web application.
In this blog, you’ll learn how to write better custom command logs in Cypress, keeping them clear and helpful during the testing process.
TABLE OF CONTENTS
Why Use Custom Commands?
When working on a medium to large web project, you write more and more tests. Then you notice that you’re copying and pasting and reusing the same piece of code everywhere. It not only makes your tests longer and harder to read but also impacts the maintainability, reusability, and readability of the command.
Custom commands allow you to bundle these repetitive tests into single reusable functions. It simplifies your tests, making them cleaner and more readable. It also allows you to add your logging messages, giving you better visibility when you perform Cypress debugging.
Here is an example of a short snippet demonstrating how you can add custom messages to Cypress logs:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
Cypress.Commands.add('addToCart', (item) => { cy.log(`Adding ${item} to cart`); cy.get(`[data-item="${item}"]`).click(); cy.get('.cart-count').should('contain', 1); cy.log(`${item} successfully added to cart`); }); describe('Cart Test', () => { it('should log custom messages when adding items to cart', () => { cy.visit('/shop'); cy.addToCart('Laptop'); }); }); |
In this snippet, messages like Adding ${item} to cart and ${item} successfully added to cart are logged using the cy.log() method.
Subscribe to the LambdaTest YouTube Channel and stay updated with more such tutorials.
How to Write Custom Commands for Cypress Logs?
When writing custom commands for Cypress logs, there are different ways we can log information during test execution, each serving different purposes depending on the level of detail.
Before we start with the examples of writing custom commands for Cypress logs, we need to install and configure Cypress in our project before running the examples. To achieve scalability and reliability, we will use cloud-based testing platforms such as LambdaTest.
LambdaTest is an AI-powered test execution platform that lets you perform automated browser testing on its Cypress cloud across 40+ browser versions. You can also run Cypress tests online in parallel to achieve faster, consistent, and reliable testing results.
Check out this documentation to get started with Cypress testing on LambdaTest.
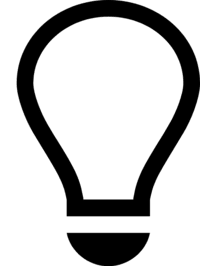
Run Cypress tests across real browsers and OSes. Try LambdaTest Today!
Prerequisites
Before we start with the examples on custom commands for Cypress logs, we need to install and configure Cypress in our project before running the examples.
- At the root of your project, add a lambdatest-config.json file for specifying environment settings, such as your LambdaTest credentials, browser configurations, and other options. In the file, paste the following code snippet:
- You can get your LambdaTest credentials from your LambdaTest Account Settings under the Password & Security tab. In the above code snippet, replace <your_lambdatest_username> and <your_lambdatest_access_key> in lambdatest-config.json with your actual credentials.
- Before running tests on LambdaTest, ensure you have the LambdaTest Cypress CLI installed in your project. You can install it using the following command:
- Once your configuration and credentials are in place, you can run your Cypress tests on LambdaTest using the following command:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
{ "lambdatest_auth": { "username": "<your_lambdatest_username>", "access_key": "<your_lambdatest_access_key>" }, "browsers": [ { "browser": "chrome", "platform": "Windows 10", "version": "latest" }, { "browser": "firefox", "platform": "macOS Big Sur", "version": "latest" } ], "run_settings": { "reporter_config_file": "base_reporter_config.json", "build_name": "build-name", "parallels": 1, "specs": "./cypress/e2e/*.spec.js", "ignore_files": "", "network": false, "headless": false, "npm_dependencies": { "cypress": "13.2.0" } }, "tunnel_settings": { "tunnel": false, "tunnel_name": null } } |
1 |
npm install -g lambdatest-cypress-cli |
1 |
npx lambdatest-cypress run |
Verbose Level Logging
By default, Cypress doesn’t natively support logging levels (such as info, warn, or error), but you can simulate verbosity in your custom commands by building your Cypress log levels.
You can implement custom verbosity levels by combining the Cypress.log() method with custom logging functions:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
function logWithLevel(level, message) { if (Cypress.env('LOG_LEVEL') === level || Cypress.env('LOG_LEVEL') === 'verbose') { Cypress.log({ name: level.toUpperCase(), message: message, }); } } // Example of a custom command using log levels Cypress.Commands.add('addToCart', (itemName) => { logWithLevel('info', `Adding ${itemName} to cart`); // Info log cy.get('[data-test="product-item"]').contains(itemName).click(); logWithLevel('success', `${itemName} added to cart successfully`); // Success log }); |
To control Cypress log verbosity during test execution, you can set the LOG_LEVEL environment variable when running your tests. For example, setting LOG_LEVEL to verbose would display all logs, while setting it to info would only show info-level messages.
You can start your test runner with the below-given command:
1 |
npx cypress run --env LOG_LEVEL=info |
This approach allows you to manage custom Cypress log levels as you see fit.
Let’s look at some other ways of logging in Cypress.
Basic Logging With cy.log()
It is one of the lightest Cypress logging mechanisms. It simply prints a message in the command log inside the Cypress Test Runner, making it easy to add clear and human-readable messages throughout the test runs.
The basic syntax for the cy.log() method is:
1 |
cy.log(“message-you-want-logged”); |
The cy.log() method accepts a string as an argument representing the message to display in the Cypress Test Runner. It can be any string or even concatenated values to make logs dynamic and descriptive.
You can also pass multiple arguments to the cy.log() method:
1 |
cy.log(message1, message2, ...); |
Each argument will be converted to a string and concatenated together to form a complete log message.
Let’s understand the cy.log() method with the help of a test scenario. For this, let’s use the LambdaTest eCommerce Playground website.
Test Scenario:
|
Implementation:
Create a loginusingcy.log.spec.js file and add the following test script. This script will handle Cypress logging into the web application and log some messages along the way. When the script is done running, it will log Login submitted.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Cypress.Commands.add("login", (username, password) => { cy.log("Starting the login process for", username); // Log message cy.get("#input-email").type(username); cy.get("#input-password").type(password); cy.get('input[type="submit"]').click(); cy.log("Login submitted"); // Log message }); describe("login lambdatest playground", () => { it("should successfully log in with valid credentials'", () => { cy.visit( "https://ecommerce-playground.lambdatest.io/index.php?route=account/login" ); cy.login("umavictor11@gmail.com", "ulgfiygfiuwef"); }); }); |
The email field is located using its ID attribute:
1 |
cy.get('#input-emaill').type(username); |
Here, #input-email is a CSS selector targeting the field with the ID of input-email.
Similarly, the password field is located using its ID attribute:
1 |
cy.get('#input-password').type(password); |
Code Walkthrough:
Use the Cypress.Commands.add() method to create a new custom command login that accepts username and password as parameters. Then, in the login command, we first log a message indicating the login process has started for the given username using cy.log(“Starting the login process for”, username).
The next step is to log into the website by entering the email and password. The email field is located using the id attribute. In the login command, we finally log a message indicating that the login form has been submitted.
The describe() block defines a test suite named login lambdatest playground. Finally in the describe block, we open the target URL using the cy.visit() method and call the login custom command we created.
Test Execution:
Shown below is the Cypress test execution screenshot on the LambdaTest Web Automation dashboard:
Here are the Cypress logs that are generated when the test runs successfully:
Advanced Logging With Cypress.log()
The Cypress.log() method is a much more advanced version of logging where you can create your custom log entries with more control. You can name a log, add your message, and even add additional properties that will show when that log entry is expanded in the Cypress Test Runner.
The basic syntax for Cypress.log() method is:
1 |
Cypress.log(options) |
Where options can be:
- name: The name of the log entry.
- message: The message to display.
- consoleProps() function: It returns an object that shows in the DevTools console.
Let’s use the same example to see how the Cypress.log() method differs from the cy.log() method.
Test Scenario:
|
Implementation:
This Cypress test script defines a custom command called login to handle the login functionality for the LambdaTest eCommerce Playground website.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
Cypress.Commands.add("login", (email, password) => { let warningMessage; const log = Cypress.log({ name: "login", message: `Logging in as ${email}`, consoleProps: () => { return { email: email, password: password, message: warningMessage, }; }, }); cy.visit( "https://ecommerce-playground.lambdatest.io/index.php?route=account/login" ); cy.get("#input-email").type(email); cy.get("#input-password").type(password); cy.get('input[type="submit"]').click(); cy.get(".alert-danger").then(($alert) => { if ($alert.length) { warningMessage = $alert.text(); log.end(); } }); }); describe("login lambdatest playground", () => { it("should successfully log in with valid credentials'", () => { cy.login("umavictor11@gmail.com", "ulgfiygfiuwef"); }); }); |
Code Walkthrough:
Create a custom log using the Cypress.log() method with specific options. Now set the name to login, the message to an array containing Logging in as user@example.com, and consoleProps() as a function that returns an object with the email and password.
Then, log into the LambdaTest eCommerce Playground by entering the email and password and locating the email field. Following this, open the target URL using the cy.visit() method and call the custom login command. When the cy.login() method is invoked, our custom Cypress.log() method logs the message Logging in as user@example.com.
Test Execution:
Shown below is the Cypress test execution screenshot on the LambdaTest Web Automation dashboard:
Here are the Cypress logs that are generated when the test runs successfully:
But what if we also want to log a value that wasn’t passed in as a parameter to our custom command. For example, when entering incorrect login details to the login form, it may be helpful to log the displayed warning message. It can be achieved by capturing the warning message within the custom command and including it in the log.
Test Scenario:
|
Implementation:
This test script defines a custom Cypress command login to automate the login process on the LambdaTest eCommerce Playground website and checks for any login error alerts.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
Cypress.Commands.add("login", (email, password) => { let warningMessage; . const log = Cypress.log({ name: "login", message: `Logging in as ${email}`, consoleProps: () => { return { email: email, password: password, message: warningMessage, }; }, }); cy.visit( "https://ecommerce-playground.lambdatest.io/index.php?route=account/login" ); cy.get("#input-email").type(email); cy.get("#input-password").type(password); cy.get('input[type="submit"]').click(); cy.get(".alert-danger").then(($alert) => { if ($alert.length) { warningMessage = $alert.text(); log.end(); } }); }); describe("login lambdatest playground", () => { it("should successfully log in with valid credentials'", () => { cy.login("umavictor11@gmail.com", "ulgfiygfiuwef"); }); }); |
Code Walkthrough:
The Cypress.log() function is set up by passing in a few options. First, the name identifies the log entry as login. Next, the message shows a custom message like Logging in as ${email} right inside the Cypress Test Runner.
Finally, consoleProps provides a function that returns an object with details such as the user’s email, password, and a placeholder for the warningMessage. When you expand the log entry in DevTools, you’ll see these extra details.
We will verify the login functionality with valid credentials. To look for a warning message, we use the cy.get() method to find any alert elements (.alert-danger). If we find one, we update our warningMessage variable with the alert’s text and then call the log.end() method to let Cypress know that our logging is done.
Backend Logging With cy.task()
The cy.task() method is one of the features for logging in Cypress. It allows running code on a Node.js backend, way outside the JavaScript sandbox of the browser. This comes in very handy in situations where something is impractical to do from the context of a browser.
For instance, interacting with the file system, accessing environment variables, or logging data that needs to be persisted or processed elsewhere, outside the scope of a test.
It can also be helpful when you want Cypress logs to be permanently available for storage in external monitoring systems or logging or to be organized in a way that does not depend strictly on how Cypress Test Runner organizes them.
We can define our tasks in the cypress.config.js file. Let’s demonstrate how we can do this by setting up backend logging using the cy.task() method.
Implementation (cypress.config.js file):
This test script defines a cypress.config.js file that sets up a custom task for logging messages to a file during testing.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 |
const { defineConfig } = require('cypress') const fs = require('fs') module.exports = defineConfig({ e2e: { setupNodeEvents(on, config) { on("task", { logToFile(message) { const fs = require("fs"); const path = require("path"); // Define the log file path const logFile = path.join( __dirname, "..", "logs", "backend-log.txt" ); // Append the message to the log file fs.appendFileSync( logFile, `${new Date().toISOString()} - ${message}\n` ); // Return null to signal that the task is complete return null; }, }); }, }, }) |
Code Walkthrough:
The on(‘task’, { … }) function in Cypress allows you to define custom tasks that can be called during test execution. One such task, logToFile(message), appends a given message to a log file using the fs module. It writes the log message to a file named backend-log.txt in a specified logs directory.
Using fs.appendFileSync(…), the task records each log message with a timestamp, providing contextual information about when each message was logged.
Implementation (in the spec file):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Cypress.Commands.add("customLogin", (username, password) => { cy.task("logToFile", `Attempting login with username: ${username}`); cy.get("#input-email").type(username); cy.get("#input-password").type(password); cy.get('input[type="submit"]').click(); cy.task("logToFile", `Login submitted for username: ${username}`); }); |
Code Walkthrough:
The cy.task(‘logToFile’, ‘…’) sends a message to the logToFile task defined in the config file. The message is logged to the backend-log.txt file. The log messages include details about the login attempt, making it easier to trace actions and debug issues after the test run.
Best Practices for Using Custom Commands
When using custom Cypress logs, a few simple tweaks can take your logging game to the next level. Here are some easy, practical tips that will help keep your logs clean and useful:
- Give your commands names that make sense right away. No one wants to guess what logInfo does, but something like logFormSubmission is much easier to understand. The goal is to make it obvious what your logs are tracking without needing extra explanation.
- Don’t hardcode things like email addresses or user data. Instead, pass them as parameters. It makes your logs reusable across different scenarios, whether it’s for a user login or a product search. It keeps your test scripts clean and adaptable.
- Using the cy.wait() method to pause for a set amount of time isn’t ideal. It slows things down unnecessarily. Instead, use the cy.intercept() method or other event-driven waiting techniques to ensure your tests are pausing only when needed, like for network responses. It’s more efficient and keeps your logs precise.
- Don’t scatter assertions everywhere. Combine related ones into a single custom log or command. It keeps your logs clean, and your tests run smoother because there’s less jumping around between different steps.
- Cypress commands are designed to chain together, so make sure your custom logs fit right into the flow. When your commands return chainable (like with the cy.wrap() method), your logs will reflect the natural flow of the test, making everything easier to read and debug.
- Whenever possible, set up your app’s state through code, not UI interactions. For example, use the cy.request() method to log in rather than filling in forms every time. It’s faster and keeps your logs focused on the important stuff.
Conclusion
In Cypress, you can make your test more readable and debuggable with different types of logging like cy.log() and Cypress.log() methods. These will help you keep track of what’s going on within your custom commands, while the console.log() method enables you to test details directly in the browser’s console.
When more advanced logging is required, the cy.task() method can log messages on the server side, which allows you to easily manage logs that may need to be stored or analyzed later. Moreover, if your software project requires even more flexibility, you can leverage third-party logging libraries to manage and administer your Cypress logs.
By thoughtfully incorporating these logging methods, you will create tests that are more reliable and easier to troubleshoot and maintain over time.
Frequently Asked Questions (FAQs)
Where are Cypress logs?
Cypress logs are usually visible in the test runner interface and can also be output to files when custom logging tasks are set up.
What is log in Cypress?
A log in Cypress refers to information output related to test execution, such as steps, assertions, or errors, to help track and debug test flows.
Citations
- Cypress.log: https://docs.cypress.io/api/cypress-api/cypress-log
Got Questions? Drop them on LambdaTest Community. Visit now