How to Use CSS Grid repeat() Function
Aakash Rao
Posted On: May 14, 2024
175245 Views
26 Min Read
As websites become more engaging and appealing with various visual layouts, creating balanced and responsive layouts is no longer a choice but a necessity. This is where CSS Grid steps in, offering a powerful grid-based system that allows the developers to arrange content in a structured and flexible manner.
CSS Grid provides a repeat() function, enabling developers to create Grid rows and column patterns efficiently. It also allows for creating responsive layouts without the necessity of media queries. With the CSS Grid repeat() function, you also adopt the idea of the “Don’t Repeat Yourself” (DRY) coding principle.
Using the CSS Grid repeat() function promotes efficiency and elegance by reducing redundancy; you save lines of code and enhance the readability and maintainability of your layout definitions.
In this blog, you will learn everything about CSS Grid repeat(), syntax, and various CSS Grid repeat() methods. You will also see how this function makes layout creation more intuitive and aligns perfectly with the core principle of avoiding unnecessary repetition.
Let’s get started!
TABLE OF CONTENTS
Understanding the Explicit vs Implicit Grid Layout
Before we head on to learning about the CSS Grid repeat() function, it’s important to know the difference between explicit and implicit grid layouts.
In CSS Grid, layouts are classified into two types: explicit and implicit. These terms refer to how the grid tracks (rows and columns) are defined and created within a grid container.
Explicit Grid Layout
The explicit grid layout refers to the defined grid structure you usually create using Cascading Style Sheets (CSS) properties like grid-template-columns and grid-template-rows. In other words, you must explicitly specify the number and size of rows and columns that the grid should have.
Here, you determine how exactly the grid should be structured, including its dimensions and layout.
1 2 3 4 5 6 |
.grid-container { display: grid; /* Explicitly defining the rows and columns */ grid-template-columns: 1fr 2fr 1fr; grid-template-rows: 100px 200px 100px 100px; } |
In the above example code, the grid-template-columns property creates three columns with relative widths, and the grid-template-rows property creates four rows with fixed heights.
See the Pen
CSS Grid repeat() Function – Explicit Grid by Aakash Rao (@aakash_codes)
on CodePen.
Output:
Implicit Grid Layout
The implicit grid layout is the part of the CSS Grid that is automatically created to accommodate additional content beyond what is explicitly defined. This is particularly useful when there are more items to place in the grid than the number of tracks that have been explicitly defined. The implicit grid adapts to the added content, automatically creating additional rows or columns as needed.
1 2 3 4 5 6 7 |
.grid-container { display: grid; grid-template-columns: 1fr 1fr 1fr; grid-template-rows: 100px 100px; /* Implicit Grid */ grid-auto-rows: 200px; } |
In the above example, the grid-auto-rows property defines the height of the rows in the implicit grid. As you have added more items (>6 items) to the grid, additional rows are created automatically, and their height has been set to 200px.
See the Pen
CSS Grid repeat() Function – Implicit Grid by Aakash Rao (@aakash_codes)
on CodePen.
Output:
A point to note about the implicit grid is that even if you don’t define the size of these implicitly created tracks using grid-auto-columns and grid-auto-rows, they will still be created, but their size will be based on the default value of auto. This might only sometimes produce the desired layout you want, as the tracks will adjust their size according to the content within them.
To learn more about CSS and CSS advanced tips and tricks, follow this guide on advanced CSS and make your website layouts look more professional.
Exploring the CSS Grid repeat() Function
The CSS Grid repeat() function creates a repeated pattern of track sizes (rows or columns) within a grid. This function defines a consistent layout with repetitive track sizes, as it helps simplify the code by reducing repetition.
The CSS Grid repeat() function takes two main arguments separated by commas: the number of repetitions and the track size.
The first value, i.e., <count>, represents the number of repetitions of how many times the track size should be repeated. This can be a <length> value, auto-fill, or the auto-fit keyword. The auto-fill and auto-fit are special keywords that help us dynamically set the number of repetitions based on the available space.
The second function value, i.e., <track>, defines the size of each track. The track can be in various units, such as:
- Length value (px) or percentages with the CSS Grid repeat() function.
- min-content keyword with the CSS Grid repeat() function.
- max-content keyword with the CSS Grid repeat() function.
- auto keyword with the CSS Grid repeat() function.
- auto-fit and auto-fill keywords with the CSS Grid repeat() function.
- Named lines with the CSS Grid repeat() function.
- fit-content() with the CSS Grid repeat() function.
- minmax() with the CSS Grid repeat() function.
- min() or max() with the CSS Grid repeat() function.
Now, as you can notice from the values of the CSS Grid repeat() function, there are many possible options for the second argument for setting the track size, and they can start to look confusing, especially when several of them are combined.
In further sections of this blog on the CSS Grid repeat() function, you will learn each argument for track size separately, try to differentiate the use cases, and understand their importance.
But before moving forward, let’s learn the typical usage of the CSS Grid repeat() function with an example where you will create a grid layout with five equal-sized columns.
Below is the code:
1 2 3 4 |
.grid { display: grid; grid-template-columns: 1fr 1fr 1fr 1fr 1fr; } |
However, to make the code more efficient and readable, you can leverage the CSS Grid repeat() function:
1 2 3 4 |
.grid { display: grid; grid-template-columns: repeat(5, 1fr); } |
In the above code example, the repeat() function defines the grid’s column structure. Specifying repeat(5, 1fr) indicates that you want five columns, each occupying an equal fraction of the available space (1fr). This concise approach achieves the same result as the longer version of the values list you saw earlier.
See the Pen
CSS Grid repeat() Function – Simple Example by Aakash Rao (@aakash_codes)
on CodePen.
Output:
The repeat() function in such scenarios allows us to specify the desired pattern in a compact and maintainable way. This pattern becomes especially advantageous as the CSS Grid Layouts become more complex or there is a need to ensure consistency across multiple parts of the CSS.
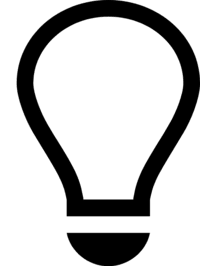
Enhance your website layouts and validate their responsiveness across 53+ device screen sizes. Try LambdaTest Today!
Now that you know some basics, let’s learn more about the CSS Grid repeat() function, exploring its various values to create the desired layout effectively.
Different Techniques of Using the CSS Grid repeat() Function
Whether setting up a simple grid for a basic webpage or constructing detailed layouts for complex web applications, understanding these techniques will enable you to harness the full potential of the CSS Grid repeat() function. This function works like magic, offering great flexibility and efficiency. With repeat(), you can create grid layouts that adapt and fit well on different screen sizes and types of content.
Length Values With the CSS Grid repeat() Function
We typically use the CSS Grid repeat() function with length values to define a consistent size for the grid tracks while repeating them across the grid container. Let’s break down how this works with a code example.
If you want to create a grid with three columns, each with a width of 200px, you want this pattern to repeat three times horizontally. Here’s how you can achieve this using the CSS Grid repeat() function:
1 2 3 4 |
.grid-container { display: grid; grid-template-columns: repeat(3, 150px); } |
In the above code example, the grid-template-columns property is set to repeat(3, 150px). The second argument, 150px, defines the size of each track for the column.
See the Pen
CSS Grid repeat() Function – Length Values (using px) by Aakash Rao (@aakash_codes)
on CodePen.
Output:
You can also set up a grid using some different length units. Let’s create one with four columns using the em unit relative to the element’s font size.
1 2 3 4 |
.grid-container { display: grid; grid-template-columns: repeat(4, 4em); } |
In the above code example, we have set the column width to 4em. If the font size of the .grid-container element is 16px (often the default), then each column will be 64px wide (4 times 16px).
See the Pen
CSS Grid repeat() Function – Length Values (using em) by Aakash Rao (@aakash_codes)
on CodePen.
Output :
Try exploring more with this example by changing the width of columns of the grid with other length unit values, such as 12ch or 25%, and so on, to see their effect in action.
min-content Keyword With the CSS Grid repeat() Function
The min-content keyword is a useful value used with the CSS Grid repeat() function to create flexible grid layouts. When you use min-content as a track size, the column or row track will be sized based on the intrinsic content of its items, essentially taking the minimum space required by the content within the track.
The min-content keyword is useful when you want to avoid unnecessarily wide empty spaces within the grid, allowing the content to dictate the width of the tracks. Let’s break this down with a code example:
Suppose you have a grid where you want the columns to adjust their width based on the content within them automatically. Here’s how you can achieve this using the min-content keyword within the CSS Grid repeat() function:
1 2 3 4 |
.grid-container { display: grid; grid-template-columns: repeat(4, min-content); } |
Here, the grid-template-columns property is set to repeat(4, min-content). This means that the grid will have four columns, and each column’s width will be determined by its content.
See the Pen
CSS Grid repeat() Function – min-content keyword by Aakash Rao (@aakash_codes)
on CodePen.
Output:
In the above output, you can notice that the second column, which includes a long text, makes the entire column wide based on the length of the text. The point to remember while using min-content is that it causes the columns to have different widths depending on their content.
max-content Keyword With the CSS Grid repeat() Function
The max-content keyword is another valuable method used with the CSS Grid repeat() function to create grid layouts that expand based on the largest item within the track. When you use max-content as a track size, the column or row track will be sized to fit the maximum content width of its items.
Let’s take an example of creating a grid where columns adjust their width to accommodate the widest content within them. You can achieve this using the max-content keyword within the CSS Grid repeat() function:
1 2 3 4 |
.grid-container { display: grid; grid-template-columns: repeat(4, max-content); } |
Here, the grid-template-columns property is set to repeat(4, max-content). This means that the grid will have four columns, and each column’s width will be determined by its content.
See the Pen
CSS Grid repeat() Function – max-content keyword by Aakash Rao (@aakash_codes)
on CodePen.
Output:
In the above output, you can notice that all the other columns adapt to the same width based on the text of the second column and the first item. This consistent width is determined by the width required to fit the longest content, Native Mobile App Testing.
As a result, all the items in that grid column adopt the same width as “Native Mobile App Testing,” ensuring a uniform and visually organized layout.
minmax() With the CSS Grid repeat() Function
The minmax() function is another powerful technique that allows developers to define a flexible range of sizes for grid tracks. It’s often used to ensure tracks stay manageable and manageable, providing a balanced and responsive layout. This function helps to create grids that can adapt well to different screen sizes and content lengths.
The minmax() function takes two arguments:
- minSize: The minSize parameter specifies the minimum size that the track can be. It can be of any CSS length unit (such as px, em, rem, etc.) or using relative units (such as fr for fractions of available space).
- maxSize: The maxSize parameter specifies the maximum size that the track can be. Similar to minSize, it can also be specified using any CSS length unit or relative unit.
When used with the CSS Grid repeat() function with minmax() it creates a pattern of tracks where each track’s size falls within the specified range. This is particularly useful for ensuring that tracks stay manageable, leading to content truncation or too large, causing unwanted empty space.
Below is an example of using minmax() with the CSS Grid repeat() function:
1 2 3 4 |
.grid-container { display: grid; grid-template-columns: repeat(3, minmax(100px, 1fr)); } |
In the above code example, the grid columns are set to have a minimum width of 100px and can expand up to take up available space (1fr). If there’s extra space beyond the 200px minimum, it will be distributed evenly among the columns.
See the Pen
CSS Grid repeat() Function – minmax() function by Aakash Rao (@aakash_codes)
on CodePen.
Output
From the above example, you can notice that the minmax() function with the CSS Grid repeat() function is incredibly helpful for creating responsive layouts that adapt well to various content lengths while maintaining a balance between track sizes.
min() or max() With the CSS Grid repeat() Function
Combining the minmax() function with the min() or max() functions within the CSS Grid repeat() function in CSS Grid provides a powerful way to create flexible and adaptive grid layouts.
In this section, let us learn how this combination works and if it’s effective.
As you saw earlier, the minmax() function defines a range of sizes for grid tracks, allowing developers to set both a minimum and maximum size.
The min() and max() functions, on the other hand, are used to calculate the minimum or maximum value from a list of values.
min() function: This function calculates the minimum value from a set of provided values or expressions. It returns the smallest value among the given list.
Sample code:
In the above code example, the width of div elements is set to the minimum value of 200px, 50% of the parent element’s width, and the width is not larger than 300px.
max() function: This function calculates the maximum value from a set of provided values or expressions. It returns the largest value among the given list.
Sample code:
In the above code example, the width of div elements is set to the maximum value of 200px, 50% of the parent element’s width, and the width is not smaller than 300px.
When used in combination with the CSS Grid repeat() function, you can create a repeating pattern of grid tracks where each track’s size is determined by the range defined using minmax() and the calculations from min() to max().
Let’s apply the min() function as the minSize value of the minmax() function, which will help us create a responsive layout:
1 2 3 4 |
.grid-container { display: grid; grid-template-columns: repeat(3, minmax(min(80px, 8vw), 1fr)); } |
In the above code example, the responsive grid layout has three columns, with a minimum width of either 80px or 8% of the viewport width (whichever is larger). The columns can expand to take up available space equally. This is useful for accommodating varying content lengths while maintaining a balanced and visually pleasing layout.
See the Pen
CSS Grid repeat() Function – minmax() function with min() or max() and repeat() by Aakash Rao (@aakash_codes)
on CodePen.
Output:
auto Keyword With the CSS Grid repeat() Function
The auto keyword in CSS Grid is used to automatically size columns or rows based on their content or intrinsic size. The auto keyword, when combined with the CSS Grid repeat() function, can help create flexible grid layouts where some tracks will have different sizes based on content while others take up a proportional amount of available space.
Let’s take an example of creating a grid with 2 column sets (a total of four columns). For each repetition, the first column will have an automatically adjusted width (auto) based on its content, and the second column will take up the remaining available space equally, using the 1fr unit.
1 2 3 4 |
.grid-container { display: grid; grid-template-columns: repeat(2, auto 1fr); } |
In the above code example, the .grid container class consists of two columns. The first column’s width is based on content, while the second column takes up the remaining space.
See the Pen
CSS Grid repeat() Function – auto keyword by Aakash Rao (@aakash_codes)
on CodePen.
Output:
Using a combination of auto and 1fr, you create a layout where the first column adjusts to accommodate its content while the second column distributes the remaining space proportionally. This technique is beneficial for balancing available space among grid items. To learn more about fr (fractional unit) in CSS, follow this guide on CSS units and get detailed insights.
auto-fit And auto-fill Keywords With the CSS Grid repeat() Function
The auto-fit and auto-fill keywords, when used with the CSS Grid repeat() function, are the most used and powerful techniques for creating flexible and responsive grid layouts. They help determine how many columns or rows should be created dynamically in a grid based on the available space and the size of the grid items.
Below, you will learn about both keywords in detail.
auto-fit Keyword
The auto-fit keyword is used within the CSS Grid repeat() function to automatically create as many tracks (columns or rows) as can fit in the container while maintaining their specified size.
In the above code example, the .grid-container class is used to apply grid layout to the web element. The CSS property grid-template-columns: repeat(auto-fit, minmax(200px, 1fr)) defines the number and size of columns in the grid.
The first argument allows the grid to automatically adjust the number of columns based on the available space. The second argument, the minmax() function, defines the size of each column. The first argument of minmax() sets the minimum width of each column to 200 px, and the second argument (1fr) allows the columns to expand and fill any remaining space equally.
If there’s extra space available, these tracks will expand to fill the space. If there’s insufficient space, the tracks will shrink or collapse accordingly.
auto-fill Keyword
The auto-fill keyword is also used within the CSS Grid repeat() function. It behaves similarly to auto-fit but with a subtle difference. The auto-fill keyword creates as many tracks as possible while attempting to maintain the specified track size.
In the above code example, the CSS property grid-template-columns defines the number and size of columns in the grid. It uses the repeat() function with auto-fill as the first argument, automatically creating as many columns as possible to fill the available space. The second argument, the minmax() function with a width of 200px, sets the minimum width of each column to 200px, while the 1fr unit allows columns to grow and fill any remaining space equally.
If there’s extra space, tracks will expand to fill the space; if there’s not enough space, the tracks will remain their specified size, potentially leaving empty space.
When using the auto-fill and auto-fit keywords with the CSS Grid repeat() function, it’s straightforward. Simply replace the number of repetitions with the keyword that suits your layout needs.
Let’s understand the usage of auto-fit and auto-fill with minmax() and try to see the difference between both with an example.
HTML:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
body> <h2>auto-fill</h2> <div class="auto-fill-container container"> <div>Online Browser Testing</div> <div>Native Mobile App Testing</div> <div>Selenium Testing</div> <div>Real Devices Cloud</div> <div>Cypress Testing</div> <div>Visual Regression Cloud</div> </div> <hr /> <h2>auto-fit</h2> <div class="auto-fit-container container"> <div>Online Browser Testing</div> <div>Native Mobile App Testing</div> <div>Selenium Testing</div> <div>Real Devices Cloud</div> <div>Cypress Testing</div> <div>Visual Regression Cloud</div> </div> </body> |
CSS:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
.auto-fill-container { grid-template-columns: repeat(auto-fill, minmax(150px, 1fr)); } .auto-fit-container { grid-template-columns: repeat(auto-fit, minmax(150px, 1fr)); } /* OTHER STYLES */ .container { display: grid; gap: 20px; } .container > div { display: flex; justify-content: center; align-items: center; background-color: white; padding: 20px; } body { font-family: "Open Sans", sans-serif; font-size: 22px; padding: 2rem; background-color: #0ebac5; color: #333; } * { margin: 0; padding: 0; box-sizing: border-box; } hr { margin-block: 80px; } |
As you can see from the above code example, the key difference between auto-fit and auto-fill keywords is how they handle empty space. The auto-fit keyword tries to fill empty space by expanding the tracks to utilize the entire container width, potentially causing them to exceed their specified size.
On the other hand, the auto-fill keyword tries to maintain the specified size for the tracks, even if it results in empty space.
See the Pen
CSS Grid repeat() Function – auto-fit / auto-fill keyword with repeat() by Aakash Rao (@aakash_codes)
on CodePen.
Output:
Both auto-fit and auto-fill are particularly useful for creating responsive grid layouts, such as flexible card grids, or for creating masonry grid structures.
Below is an example of a Masonry grid layout leveraging the CSS Grid repeat() function using the auto-fit keyword and minmax() function.
See the Pen
CSS Grid repeat() Function: Masonry Image Gallery by Aakash Rao (@aakash_codes)
on CodePen.
Output:
Now, you also have the option to make use of the min() or max() function inside the minmax() function, with auto-fit or auto-fill keywords to achieve even more dynamic and responsive grid layouts.
You can create other layouts, such as the Masonry grid layout in CSS Grid. To explore different layout options and make your website look presentable and responsive, follow this guide on CSS Grid Layout generators.
Named Lines With the CSS Grid repeat() Function
Named lines in CSS Grid allow us to assign names to lines within the grid, such as column and row lines, which can then be referenced in the grid’s properties. The CSS Grid repeat() function can be combined with named lines to create more structured and readable grid layouts.
It provides human-readable labels to the lines in the grid, making it easier to reference and position items within the grid. You can name both column and row lines.
Here’s an example of naming column lines,
HTML:
1 2 3 4 5 6 7 8 9 10 11 12 |
<div class="magazine"> <div class="article-tech">Tech Article</div> <div class="article-content">Content Article</div> <div class="article-lifestyle">Lifestyle Article</div> </div> |
CSS :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
.magazine { display: grid; grid-template-columns: [start-tech] 1fr [end-tech start-content] 2fr [end-content start-lifestyle] 1fr [end-lifestyle]; } .article-tech { grid-column: start-tech / end-content; background-color: #ffd700; } .article-content { grid-column: start-content / end-lifestyle; background-color: #87ceeb; } .article-lifestyle { grid-column: start-lifestyle / end-lifestyle; background-color: #90ee90; } |
See the Pen
CSS Grid repeat() Function – Named Lines by Aakash Rao (@aakash_codes)
on CodePen.
Output:
Let’s explore an example of using the Named lines with the CSS Grid repeat() function.
HTML:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
<div class="container"> <div>Online Browser Testing</div> <div>Native Mobile App Testing</div> <div>Selenium Testing</div> <div>Real Devices Cloud</div> <div>Cypress Testing</div> <div>Visual Regression Cloud</div> <div>Playwright Testing</div> <div>Test Intelligence</div> <div>HyperExecute</div> <div>Automation Testing Cloud</div> <div>On-Premise Selenium Grid</div> <div>Smart TV Testing Cloud</div> </div> |
CSS:
1 2 3 4 5 |
.container { display: grid; grid-template-columns: [start] auto repeat(3, [ham] 1fr [burger]) [sidebar] 250px [end]; } |
In the above code example, we used Named grid lines to simplify content alignment in a CSS Grid layout. Named lines, designated with custom names like [ham] and [burger], act as markers for column positions. This removes the need to rely on numerical indices, making the layout more intuitive.
The CSS Grid repeat() function streamlines the creation of repetitive column patterns. In the code, repeat(5, [ham] 1fr [burger]) establishes a pattern of 3 columns, each controlled by the named lines. This combination of named lines and repeat() function offers a structured and adaptable approach to creating dynamic grid layouts in CSS.
See the Pen
CSS Grid repeat() Function – Named Lines with repeat() by Aakash Rao (@aakash_codes)
on CodePen.
Output:
fit-content() With the CSS Grid repeat() Function
The fit-content() function calculates the minimum size needed to accommodate the content within an element. It takes a single argument; if the content’s size is larger than the argument, the element will expand up to the specified maximum size. It is a valuable technique for creating grid layouts that adapt to the size of their content while maintaining a structured and organized design.
This is particularly useful for scenarios where you want the grid to be responsive to varying content lengths but within a certain range of sizes.
1 2 3 4 |
.grid-container { display: grid; grid-template-columns: repeat(3, fit-content(200px)); } |
In the above code example, the grid-template-columns property uses the CSS Grid repeat() function to create three columns, and each column’s width is determined by the fit-content(200px) function. The fit-content() function calculates the minimum size required to contain the content within an element.
In this case, fit-content(200px) indicates that each column should be wide enough to accommodate its content but not exceed a maximum width of 200px. If the content is smaller than 200px, the column will adjust to fit the content. If the content exceeds 200px, the column will cap its width at 200px.
See the Pen
CSS Grid repeat() Function – fit-content() function with repeat() by Aakash Rao (@aakash_codes)
on CodePen.
Output:
In addition to using the fit-content(), you can also prefer using CSS Flexbox to create flexible and responsive layouts. CSS Flexbox offers a simpler and more efficient way to create one-dimensional layouts, making it a valuable addition to your web development toolkit.
To learn more about the differences between CSS Grid and Flexbox, follow this guide on the key differences between CSS Grid vs Flexbox. This guide will help you understand how to use each CSS technique efficiently and how they contribute to building responsive website layouts quickly.
When designing a website layout with HTML and CSS, ensuring its responsiveness is crucial. Suppose the layout isn’t responsive to different screen sizes. In that case, users may have difficulty viewing the website on various devices, potentially leading to users leaving your site, and the organization may also face financial loss. Therefore, a well-designed, presentable, and easily navigable website attracts and retains users.
To manage the consistency and to ensure your website’s responsiveness across different devices, you can perform visual testing, UI testing, and responsive testing. This helps validate the UI alignment and ensures that your website looks and functions correctly regardless of the device viewport; you can also use tools like LT Browser to help you ensure how your website looks and works on various devices and screen sizes and help build a user-friendly website for better user experience.
LT Browser is a tool offered by LambdaTest, an AI-powered test orchestration and execution platform that lets you run manual and automated tests at scale with over 3000+ real devices, browsers, and OS combinations.
It offers a comprehensive preview of your website on various mobile devices. With over 53+ device viewports available, you can compare them side by side and synchronize interactions like scrolling, clicking, and navigation. It provides pre-installed viewports for mobiles, tablets, desktops, and laptops.
Watch the video tutorial below to become acquainted with the features and functionalities of LT Browser.
Subscribe to the LambdaTest YouTube Channel and get the latest updates on various automation testing tutorials covering Selenium testing, web device testing, cross-device testing, browser compatibility, and more.
Wrapping Up
This guide explored how the CSS Grid repeat() function is a powerful technique for creating versatile grid layouts that adapt to various design requirements and screen sizes. You now know how to create efficient and responsive grid structures using the techniques discussed.
The flexibility of the CSS Grid repeat() function allows developers to define dynamic grid patterns, efficiently managing column and row repetitions while keeping the codebase clean and concise. This is especially useful for designing complex layout structures accommodating different amounts of content.
Combining minmax() with repeat() allows you to set precise track size ranges, balancing content accommodation and space optimization. Using keywords like auto-fit and auto-fill with the CSS Grid repeat() enables us to create grids that automatically adjust to available space, ensuring a seamless user experience.
In summary, these techniques are valuable additions to our web development toolbox. As you practice and refine the skills with these techniques, you can excel in each project’s grid layout to its specific needs, using the CSS Grid repeat() function in conjunction with other CSS tricks.
So, keep exploring, learning, and designing with confidence!
Frequently Asked Questions (FAQs)
Can I use the repeat() function for rows and columns in the same grid layout?
Surely yes! The CSS Grid repeat() function is versatile and can define rows and columns in a grid layout. You can apply it to either grid-template-columns or grid-template-rows (or both) to create a balanced and organized grid structure.
This is particularly handy when you want to establish a consistent layout for both the horizontal and vertical aspects of your design.
How does the auto-fit keyword differ from auto-fill when combined with repeat()?
Both auto-fit and auto-fill work with the CSS Grid repeat() function to create flexible grid layouts. However, there’s a subtle difference between the two. When auto-fit is used, the grid tracks will expand to fill the available space, potentially creating extra space if the items don’t completely fill the container.
On the other hand, with auto-fill, the tracks maintain their specified size, which might lead to empty spaces if items can’t fully utilize the tracks. Choosing between them depends on whether you want to prioritize filling space (auto-fit) or maintaining consistent track sizes (auto-fill).
Can I use the minmax() function with other CSS properties besides repeat()?
Absolutely! While the minmax() function is often used within the CSS Grid repeat() function to define track size ranges, you can also use it with other CSS properties. For example, you can use it to directly set an element’s minimum and maximum size in properties like width and height and even in the Flexbox layouts.
This provides a powerful way to ensure elements adapt smoothly to different content lengths or screen sizes, enhancing the responsiveness of the design.
Got Questions? Drop them on LambdaTest Community. Visit now