How to Handle Dropdowns Using the Cypress .select() Command
Alex Anie
Posted On: March 4, 2025
147881 Views
11 Min Read
Dropdowns can be challenging to handle due to dynamic options, inconsistent values, or differences between displayed text. In Cypress, you can overcome this challenge using the .select() command.
It allows you to handle (or select) dropdowns by visible text, value, or index. The Cypress .select() command is a built-in function that interacts with and performs tests on the selected elements in a web application.
In this blog, you will learn everything you need to know about using the Cypress .select() command.
TABLE OF CONTENTS
What Is Cypress .select() Command?
The Cypress .select() command is a built-in function that is used to select a <option> tag within the <select> WebElement. It retrieves the selected <option> tags and performs tests on them.
1 2 3 4 |
.select(value) .select(values) .select(value, options) .select(values, options) |
The Cypress .select() command takes in two arguments, the first being value, which can be any of the following; value, index, or text content of the <option> tag.
The second argument is optional but can be specified as a configuration object. For example, (force: true) can be used to undo the default behavior of the .select() command.
HTML:
1 2 3 4 5 |
<select> <option value="one">apples</option> <option value="two">oranges</option> <option value="three">bananas</option> </select> |
JavaScript:
1 |
cy.get('select').select(0).should('have.value', 'one') |
Here, we get the <select> WebElement and pass in an integer with a value of zero; then, we assert that the selected WebElement should have a value of “one” as a means of validation before test execution.
The Cypress .select() command is used to handle both types of dropdowns: static and dynamic.
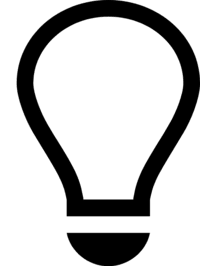
Test dropdowns with Cypress across 50+ browser versions. Try LambdaTest Today!
How to Handle Static Dropdowns?
Static dropdowns are a type of select control in which the selection content is loaded in the browser as soon as the page loads. They are usually hard-coded into the HTML of the web page.
There are different ways to select static dropdown options using the Cypress .select() command, including selecting by index, value, or text.
Select by Index
Select by index is one of the methods that can be used to select <option> tag within the <select> element. The index refers to an integer that represents the number of <option> tags within the parent <select> element. The first element is identified as 0, and then the second as 1, and so on.
To see this in action, let’s use the LambdaTest eCommerce Playground.
- Open the LambdaTest eCommerce Playground on your web browser.
- Press the Tab with F12 on your keyboard to launch the DevTools.
1 |
#entry_212434 #input-sort-212434 |
Copy the above CSS selector and press CTRL + F. Then, paste the CSS selector in the find box indicated by the red box.
This should target and select the right WebElement as highlighted in the yellow color below:
After identifying the selected element, run the Cypress test script below to execute the test case:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
describe('Select the index', () => { it('select the option tag with an of 1', () => { cy.visit('https://ecommerce-playground.lambdatest.io/index.php?route=product/manufacturer/info&manufacturer_id=9') cy.get('#entry_212434 #input-sort-212434').select(1) // Wait for the page to update or stabilize cy.url().should('include', 'sort=order_quantity') // Assert the selected option's text is 'Best sellers' cy.get('#entry_212434 #input-sort-212434 option:selected') .should('have.text', 'Best sellers') }) }) |
Your test run should be indicated as shown below:
In the image above, the Best Sellers option is selected in the select control.
Select by Value
Selecting a dropdown by value is very similar to selecting an element by index. Instead of passing integers as value to the .select() command, the value property of the <option> tag is used instead.
1 2 3 4 5 6 7 |
describe('Select web element by value', () => { it('Select the option element by the value attribute', () => { cy.visit('https://ecommerce-playground.lambdatest.io/index.php?route=product/manufacturer/info&manufacturer_id=9') cy.get('#entry_212434 #input-sort-212434') .select('https://ecommerce-playground.lambdatest.io/index.php?route=product/manufacturer/info&manufacturer_id=9&sort=p.viewed&order=DESC') }) }) |
The code above used the .select() command to interact with the <select> WebElement and retrieve the <option> tag with the value Popular text.
Here, the <select> WebElement uses the value to choose the Popular option, which corresponds to the text displayed in the dropdown. The test simulates selecting the most popular items on the Canon product list by interacting with the dropdown element.
Select by Text
To select an element by text, the .select() command received the text content as an argument.
1 2 3 4 5 6 7 |
describe('Select web element by Text', () => { it('Select the option tag with a Newest', () => { cy.visit('https://ecommerce-playground.lambdatest.io/index.php?route=product/manufacturer/info&manufacturer_id=9') cy.get('#entry_212434 #input-sort-212434') .select('Newest') }) }) |
Here, the text content of the <option> element Newest is passed to the .select() command. This allows Cypress to retrieve the WebElement associated with Newest and then perform a test on it.
From the image above, the select control selected the <option> element with the text content of the Newest element.
How to Handle Dynamic Dropdowns?
Dynamic dropdowns are selection controls in which the options are rendered dynamically depending on the content or user search input.
Initially, the <option> elements are not present when the page first loads. Instead, the dropdown waits for the user’s input or an event to trigger before it loads the options dynamically into the page.
Cypress sends requests by passing text into the input control. It then waits for the content to load on the page before interacting with it.
To handle dynamic dropdowns in Cypress, you can use .contains() and .each() commands.
.contains() Command
The .contains() command in Cypress retrieves the DOM element containing the text passed in. It can take more than one argument.
Syntax:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
.contains(content) .contains(content, options) .contains(selector, content) .contains(selector, content, options) // getting web elements that contain cy.contains(content) cy.contains(content, options) cy.contains(selector, content) cy.contains(selector, content, options) // usage cy.get('.nav').contains('About') cy.contains('Hello') |
To demonstrate, we will leverage the Cypress .contains() command to dynamically interact with dropdowns on a web page.
We will use the LambdaTest eCommerce Playground. When a user inputs “iPhone” into the navigation search bar, a list of matching product items is displayed as clickable links within the dropdown.
The Cypress .contains() command is used to assert that the search query is present within the returned product list and, if found, triggers a click action on the corresponding item. This approach is particularly useful for automating the testing of dynamic dropdowns and validating that search functionality behaves as expected.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
describe('Dynamic Dropdown Test', () => { it('should select a specific option from a dynamically loaded dropdown', () => { // Step 1: Visit the page cy.visit('https://ecommerce-playground.lambdatest.io/index.php?route=common/home'); // Replace with your actual page URL // Step 2: Click on the dropdown to trigger the loading of dynamic options cy.get('#entry_217822 > div input[placeholder="Search For Products"]').click(); // Adjust selector for the dropdown // Step 3: Wait for the dropdown options to be visible (if required) cy.get('#entry_217822 > div input[placeholder="Search For Products"]') // Selector for the dropdown options container .should('be.visible'); // Step 4: Optionally filter options by typing in the dropdown's input field cy.get('#entry_217822 > div input[placeholder="Search For Products"]').type('iPod Touch'); // Adjust if needed for input // Step 5: Select an option dynamically cy.contains('#search > div.search-input-group.flex-fill > div.dropdown > ul li', 'iPod Touch') // Adjust selector for dropdown item .click(); // Step 6: Assert that the correct option is selected cy.get('#entry_217822 > div input[placeholder="Search For Products"]').should('have.value', 'iPod Touch'); }); }); |
Output:
.each() Command
The .each() command in the dynamic dropdown iterates through the elements that match the specified text and provides an interface to interact with the selected WebElement.
Syntax:
1 2 3 4 5 6 7 8 9 10 |
cy.get('ul>li').each(($el, index, $list) => { // $el is a wrapped jQuery element if ($el.someMethod() === 'something') { //Wrap this element so we can // use cypress commands on it cy.wrap($el).click() } else { // do something else } }) |
Here, the below test script loads the specified web address and retrieves the DOM element of the input tag on the navbar.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
describe('Dynamic Dropdown Test', () => { it('should select a specific option from a dynamically loaded dropdown', () => { // Step 1: Visit the page cy.visit('https://ecommerce-playground.lambdatest.io/index.php?route=common/home'); // Replace with your actual page URL // Step 2: Click on the dropdown to trigger the loading of dynamic options and type the text "iPod" cy.get('#entry_217822 > div input[placeholder="Search For Products"]').click().clear().type('iPod'); // Step 4: iterate over the the returned nodelist and check if "Nano" text exit and click it if Yes cy.get('#search > div.search-input-group.flex-fill > div.dropdown > ul li').each(($el, index, $list)=>{ cy.log($el.text()) if($el.text() === "Nano"){ cy.wrap($el).click() } }) }); }); |
It clicks on the input tag and types the iPod text. Then it iterates over all the dynamic dropdowns and checks if the element that contains iPod matches the content Nano, and if this is true, a click operation is performed.
Advanced Use Cases of Cypress .select() Commands
When handling dropdowns, some WebElements are hidden or disabled by default, making them difficult to retrieve with the .select() command in Cypress.
Let’s look at how to handle disabled and hidden dropdowns.
Disabled Dropdowns
Disabled dropdowns are select controls set to disable using the disabled property in HTML, or :disabled CSS pseudo-class. Disabled elements prevent users from interacting with the specified WebElement.
When elements are set to disabled, it stops functioning as usual and does not respond to mouse input, keyboard events, or any other user-stimulated interaction. However, when using the Cypress .select() command, disabled elements are, by default, turned off as false. This means it cannot be selected with the Cypress .select() command.
To bypass this, the Cypress .select() command provides a second argument called force object. The force object takes in a boolean value as true to enable Cypress to select the disabled element on the DOM.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<section class="iphones" id="iphones"> <h1>Types of iPhone</h1> <section id="iphone-versions"> <label for="select-version">Choose an iPhone:</label> <select name="iPhones" id="select-version" disabled> <option value="">--Please choose an option--</option> <option value="iPhone-8">iPhone 8</option> <option value="iPhone-XR">iPhone XR</option> <option value="iPhone-11" >iPhone 11</option> <option value="iPhone-12">iPhone 12</option> <option value="iPhone-13">iPhone 13</option> <option value="iPhone-14">iPhone 14</option> </select> </section> </section> |
Here, we have a select WebElement with a specified disabled element to prevent user interactions.
The <select> WebElements with a disabled property specified, can not be accessed by Cypress. This is the default behavior in Cypress to fix this.
The Cypress .select() command is set to receive a second argument as {force: true}. When the {force: true} property is applied to an element, Cypress interacts with the element directly without enabling it or removing the disabled property in order to perform a test on it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
describe('Force Select an Element', () => { it('Force select a disabled element', () => { cy.visit('https://cdpn.io/pen/debug/MYgXLQy?authentication_hash=LQkExWmOvBxA') cy.get('#iphone-versions #select-version') .select('iPhone 11', {force: true}) .invoke('val').should('eq', 'iPhone-11') }) }) |
From the code above, the .select() command received a second argument as force and set its value to true. This enables the Cypress .select() command to interact with the disabled element while it’s still disabled or hidden.
Output:
Hidden Dropdowns
Hidden WebElements are HTML elements styled with display: none in CSS, making them invisible on the web page.
In Cypress, hidden elements are typically disabled from interaction. However, to interact with a hidden element, you can use the {force: true} option, allowing Cypress to forcefully interact with the specified element.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<section class="mac-book" id="mac-book"> <h1>Mac Book models released</h1> <section id="mac-book-models"> <label for="select-version">Choose a Mac Book:</label> <select name="mac-book" id="select-version" style="display: none;"> <option value="">--Please choose an option--</option> <option value="iMac-Pro">iMac Pro (27-inch)</option> <option value="MacBook-Air">MacBook Air (Retina, 13-inch)</option> <option value="Mac-Pro" >Mac Pro</option> <option value="Mac-mini">MacBook Pro</option> <option value="Mac-Studio">Mac Studio</option> <option value="MacBook-Air">MacBook Air</option> </select> </section> </section> |
The code above demonstrates a <select> element with its display property set to none, which hides both the <select> element and its child <option> tags, preventing them from appearing on the web page.
Here, the <select> element is set to display: none, which automatically removes the element from the DOM. In this case, the element becomes hidden from users and, as a result, stops Cypress from interacting with it.
To stop the behavior, {force: true} option is set to the .select() command, allowing Cypress to interact with the hidden element.
1 2 3 4 5 6 7 8 9 10 11 12 |
describe('Force Select an Element', () => { it('Force select a hidden element', () => { cy.visit('https://cdpn.io/pen/debug/dPbjbwK?authentication_hash=nqAwvJdQxbWr') cy.get('#mac-book-models #select-version') .select('Mac Studio', {force: true}) .invoke('val').should('eq', 'Mac-Studio') }) }) |
Output:
Select Multiple Elements
The Cypress .select() command can simultaneously select more than one <option>. This is very useful as it lets you perform multiple test cases on numerous <option> WebElements.
To interact with multiple elements at once, the Cypress .select() command accepts additional arguments in the form of multiple values. These values correspond to the text content of the <option> tags that Cypress needs to select.
1 2 3 4 5 6 7 8 9 10 |
describe('Select multiple element', () => { it('Select more than one option web element', () => { cy.visit('https://ecommerce-playground.lambdatest.io/index.php?route=product/manufacturer/info&manufacturer_id=9') cy.get('#entry_212434 #input-sort-212434') .select(['Default'], ['Best sellers'], ['Popular']) }) }) |
Output:
The Cypress .select() command selects the options passed as an argument starting from the Default option.
The above Cypress tests for handling dropdowns are run on the local grid. However, for better scalability and reliability, you can harness the capabilities offered by cloud grids such as LambdaTest.
LambdaTest is an AI-native test execution platform that lets you run tests on the Cypress cloud grid. It offers scalability, intelligent debugging, and AI-driven optimizations to streamline test automation.
Conclusion
The Cypress .select() command is a built-in function that interacts and runs tests on the <select> WebElement and dynamic dropdown in the web application. The <select> WebElement or dynamic dropdown are web controls that provide a menu or list of options for users on the web.
The .select() command can interact with dropdowns by their indexes, values, or text content. It can also run tests on multiple WebElements. However, to perform dynamic selection, more advanced techniques are required, such as .contains() and .each() Cypress commands.
Frequently Asked Questions (FAQs)
How to use select in Cypress?
You can use the .select() command to choose an option from a dropdown by its visible text, value, or index.
How do I select a file in Cypress?
To select a file in Cypress, you can use the .selectFile() command on a file input element to simulate file uploads.
How to select text in Cypress?
Cypress doesn’t have a direct text selection command, but you can simulate it using mouse events.
How do you select specific elements in Cypress?
You can use the .get() command with CSS selectors or the .contains() command to find elements based on text content.
Citations
- Cypress .select() command: https://docs.cypress.io/api/commands/select
Got Questions? Drop them on LambdaTest Community. Visit now