How to Use Selenium Click Commands Using Python
Paulo Oliveira
Posted On: July 18, 2024
523151 Views
20 Min Read
Automating mouse clicks is essential for browser automation, allowing testers and developers to simulate real user interactions on web pages. Selenium, a powerful web automation tool combined with Python, offers a robust framework for automating these interactions. By automating Selenium click commands with Python, you can streamline testing processes, enhance accuracy, and save time by effortlessly executing repetitive tasks.
TABLE OF CONTENTS
What Are Selenium Click Commands?
The Selenium click command automates your web application’s UI. Some of the Selenium click commands include:
- click(): Performs a click operation on an element.
Syntax:click(element)
- click_and_hold(): Acts as holding the left mouse button to an element.
Syntax:click_and_hold(element)
- context_click(): Performs a right-click operation on an element.
Syntax:context_click(element)
- double_click(): Performs a double-click operation on an element.
Syntax:double_click(element)
- drag_and_drop(): It holds the left mouse button on the source element. Then, it moves to the target element and finally releases the mouse button.
Syntax:drag_and_drop(element, target)
- drag_and_drop_by_offset(): Description: It holds the left mouse button on the source element. Then, it moves to the target offset and finally releases the mouse button.
Syntax:
drag_and_drop_by_offset (element, xoffset, yoffset)
- move_by_offset(): Performs movement of the mouse to an offset from the present position of the mouse.
Syntax:move_by_offset(xoffset, yoffset)
- move_to_element(): Performs mouse movement to the middle of the element.
Syntax:move_to_element(element)
- move_to_element_with_offset(): Performs mouse movement by offsetting the element specified on the page. The offsets are for the top left corner of the element.
Syntax: move_to_element_with_offset(element, xoffset, yoffset)
These commands, provided by Selenium WebDriver, help simulate Selenium click operations on WebElements like buttons, links, and more. However, you can also perform complex actions like drag and drop, click and hold, etc., using Selenium’s ActionChains library, thus enhancing your web automation scripts with precise and efficient interactions.
What Is ActionChains?
ActionChains is a Python library that automates special interactions such as context click, double click, drag and drop mouse movements, and key down and up actions. It is very useful for executing complex actions using the keyboard and mouse.
To use the ActionChains library, you need to create an ActionChains object that can call methods to perform some actions sequentially, one by one. The called actions are pushed into a queue, and when calling the “perform” method, they are fired and performed in the same order in which they were queued.
Syntax:
First, we want to show you how to create an object using the ActionChains library.
1 2 3 4 |
//import Action chains from selenium.webdriver.common.action_chains import ActionChains //create action chain object action = ActionChains(browser); |
To use the ActionChains library, import it and instantiate an object by passing the browser element where actions will be executed. ActionChains supports various mouse actions, which we will learn further in this blog on Selenium click.
On a side note, you can further enhance your Selenium automation testing by leveraging AI testing agents like KaneAI.
KaneAI is a GenAI native test assistant for high-speed quality engineering teams. With its unique AI-driven features for test authoring, management, and debugging, KaneAI allows teams to create and evolve complex test cases using natural language.
How to Perform Selenium Clicks?
You can perform mouse actions on a web page using various Selenium click commands. In this section, we will learn about different Selenium click commands with demonstrations, each executed on a cloud-based platform.
Before learning to implement the various Selenium click commands, you must gather the required libraries and necessary development tools.
Prerequisites:
To start the coding, you must follow the steps to set up Python, Selenium, and Visual Studio Code.
- First, you must download and install Python according to your operating system.
- Selenium WebDriver can be installed simply using the pip install manager. PIP is a package management system used to install/manage packages and libraries written in Python. Use the following command before installing Selenium WebDriver.
- Download and install the Visual Studio Code, one of the most used IDEs. For the coding demonstration, we will be using Visual Studio Code. You can use your preferred IDE;
1 |
pip install selenium |
Quick Note: ActionChains is installed within the Selenium WebDriver library, so you just need to use it.
Executing multiple tests in parallel on a local machine can be challenging due to limited resources and a lack of proper infrastructure. Using a cloud-based platform provides a ready-to-use test infrastructure, eliminating the need for frequent environment setups.
One such cloud platform is LambdaTest, an AI-powered test execution platform that provides a stable test infrastructure, allowing you to run manual and automated tests at scale with over 3000+ real devices, browsers, and OS combinations.
Once you have downloaded the required libraries and IDEs, you must use the capabilities to configure the environment where the test will run to use the LambdaTest cloud grid in your code. These capabilities will enable you to select the browser, browser version, and OS, capture logs, record tests, and more during the test execution.
Follow the steps below to get started with LambdaTest:
- Create an account on the LambdaTest platform.
- Obtain your LambdaTest Username and Access Key: Navigate to Profile > Account Settings > Password & Security tab.
- Update the required capabilities: Use the LambdaTest Capabilities Generator to select and generate the necessary code for the desired capabilities.
By following these steps, you’ll be set up to run your tests on LambdaTest with the appropriate configurations.
We are now prepared to execute various mouse click methods. To perform each mouse action method, we will use Selenium 4 on Windows 11, executing the tests on the latest version of the Chrome browser with a resolution of 1024×768.
We will further learn how to automate Selenium click commands, each based on specific test scenarios.
Below are the supported Selenium click commands that help you deal with various mouse operations:
Performing Single Click in Selenium
In this section, we will learn how to implement the click() method in Selenium Python to perform a single click.
To better understand the workings of the Selenium click() method, let’s take a test scenario to demonstrate its functionality.
Test Scenario:
|
You can view the page where we will automate some actions, as shown below.
Code Implementation:
Below is the code implementation of the above test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
from selenium.webdriver.common.by import By from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Key" gridUrl = "hub.lambdatest.com/wd/hub" lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/simple-form-demo") #locating the element message_field = browser.find_element(By.ID, value="user-message") button = browser.find_element(By.ID, value="showInput") your_message = browser.find_element(By.ID, value="message") # create action chain object actions = ActionChains(browser) actions.click(on_element=message_field) actions.send_keys("Hello!") actions.click(on_element=button) actions.perform() #asserting that the message presented is Hello! assert your_message.text == "Hello!" #closing the browser browser.quit() |
Code Walkthrough:
In the above code, you must locate the form’s message field, the button, and the “your message” area. These fields can be located by ID, making the code simpler.
To perform the click() operation, you must create an ActionChains object to interact with elements.
The first action will be to click on the message field for which you must use the click() method, and the on_element parameter should reference the message_field variable.
The second action will be to type ‘Hello!’ into the field. You can do this using the send_keys method.
The third step will be to click on the button.
Finally, you must call the perform() method.
To finish, you can check if your presented message on the right side of the form is “Hello!”.
Finally, you call browser.quit() to close the browser instance.
Before we learn other Selenium click methods, the code for locating the message field in the form, initializing the browser, sending keys and assertions, using the perform() method, and quitting the browser remains unchanged. Hereafter, we will only highlight the code for performing specific Selenium click commands for mouse actions.
Performing Click and Hold in Selenium
Let’s learn how to implement Selenium click_and_hold() and release() methods using Python.
Here, we will use the Selenium click command to click on a WebElement in the DOM, hold it, and then release it. This involves using the click_and_hold(element) and release() methods of ActionChains.
To better understand the workings of the click_and_hold(element) and release() method, let’s take a test scenario to demonstrate its functionality.
|
You can view the page where we will automate some actions, as shown below.
Code Implementation:
Below is the code implementation of the above test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
from selenium.webdriver.common.by import By from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Key" gridUrl = "hub.lambdatest.com/wd/hub" lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/simple-form-demo") #locating the element message_field = browser.find_element(By.ID, value="user-message") button = browser.find_element(By.ID, value="showInput") your_message = browser.find_element(By.ID, value="message") # create action chain object actions = ActionChains(browser) actions.click(on_element=message_field) actions.send_keys("Hello!") actions.click_and_hold(button) actions.release() actions.perform() #asserting that the message presented is Hello! assert your_message.text == "Hello!" #closing the browser browser.quit() |
Code Walkthrough:
The code that is responsible for performing click_and_hold() is given below.
Once you perform the click_and_hold() method, you can release() the mouse action using the release() method.
Finally, you must call the perform() method to help you perform your code instructions and then use the browser.quit(), close the browser instance.
Performing Context Click in Selenium
Let’s learn how to implement the Selenium content_click() method using Python.
Here, we will use the Selenium click command to right-click on a WebElement in the DOM. This involves using the context_click(element) method of ActionChains.
To better understand the workings of the context_click(element) method, let’s take a test scenario to demonstrate its functionality.
Test Scenario:
|
You can view the screenshot of the page below, where we will automate context click action.
Code Implementation:
Below is the code implementation of the above test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
from selenium.webdriver.common.by import By from selenium import webdriver from selenium.webdriver.common.keys import Keys from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Key" gridUrl = "hub.lambdatest.com/wd/hub” lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/simple-form-demo") #locating the element message_field = browser.find_element(By.ID, value="user-message") button = browser.find_element(By.ID, value="showInput") your_message = browser.find_element(By.ID, value="message") # create action chain object actions = ActionChains(browser) actions.click(on_element=message_field) actions.context_click() actions.perform() #closing the browser browser.quit() |
Code Walkthrough:
As you can see, the code remains the same; the only action will be to click the right mouse button and type “Hello!” We can do this in the field using the context_click() method.
Finally, you must call the perform() method to help you perform your code instructions and then use the browser.quit() to close the browser instance.
Performing Double Click in Selenium
Let’s learn how to implement the Selenium double_click() method using Python.
Here, we will use the Selenium click command to double-click on a WebElement in the DOM. This involves using the double_click(element) method of ActionChains.
To better understand the workings of the double_click(element) method, let’s take a test scenario to demonstrate its functionality.
Test Scenario:
|
You can view the screenshot of the page below, where we will automate the double-click action.
Code Implementation:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
from selenium.webdriver.common.by import By from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "your lambdatest username" accessToken = "your lambdatest access key" gridUrl = "hub.lambdatest.com/wd/hub" lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/table-sort-search-demo") #locating the element age_sorting = browser.find_element(By.XPATH, value="//th[4]") # create action chain object actions = ActionChains(browser) actions.double_click(age_sorting) actions.perform() #asserting that the first two values are 66 first_age = browser.find_element(By.XPATH, value="//tr[1]//td[@class='sorting_1']") second_age = browser.find_element(By.XPATH, value="//tr[2]//td[@class='sorting_1']") assert first_age.text == "66" assert second_age.text == "64" #closing the browser browser.quit(); |
Code Walkthrough:
The first part of the script will be the necessary code to open the desired web page, as shown in the previous section of this blog on automating Selenium click commands using Python.
Then, you need to locate the element we will double-click at. In this scenario, we will use the Age column title.
First, import the By method from selenium.webdriver.common.by.
Then, you should locate the element using XPath. You can see the information on page elements and find the Age column title element.
The element is shown in the details below. We can locate this element using the
The code for locating the element is shown below.
<th class=”sorting” tabindex=”0″ aria-controls=”example” rowspan=”1″ colspan=”1″ aria-label=”Age: activate to sort column ascending” style=”width: 81.3px;”>Age</th>
Then, use the ActionChains method double_click(), specifying the element to be double-clicked and stored in the age_sorting variable.
To check if, after the double click, the first two lines of the table have 66 as the age, we should locate the age in the first two lines using XPath again.
Then, we can finally assert that first_age equals “66” and second_age equals “64”.
Finally, you must call the perform() method to help you perform your code instructions and then use the browser.quit(), close the browser instance.
Performing Drag and Drop in Selenium
Let’s learn how to implement the Selenium drag_and_drop() method using Python.
Here, we will use the Selenium click command to drag a WebElement in the DOM to a specific target element. This involves using the drag_and_drop(element, target) method of ActionChains.
To better understand the workings of the drag_and_drop(element, target) method, let’s take a test scenario to demonstrate its functionality.
Test Scenario:
|
You can view the screenshot of the page below, where we will automate drag and drop action.
Code Implementation:
Below is the code implementation of the above test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
from selenium.webdriver.common.by import By from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Key" gridUrl = "hub.lambdatest.com/wd/hub" lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/drag-and-drop-demo") #locating the element draggable = browser.find_element(By.XPATH, value="//p[normalize-space()='Drag me to my target']") droparea = browser.find_element(By.XPATH, value="//div[@id='droppable']") # create action chain object actions = ActionChains(browser) actions.drag_and_drop(draggable, droparea) actions.perform() #asserting that when dropping, "Dropped!" is shown inside the droparea message = browser.find_element(By.XPATH, value="//div[@id='droppable']/p") assert message.text == "Dropped!" #closing the browser browser.quit() |
Code Walkthrough:
In this test scenario, we must locate the draggable WebElement and the drop area, another WebElement. We can use XPath again and store the element in “draggable” and “droparea” variables.
To drag and drop the element, you must call the drag_and_drop() method, passing the “draggable” and “droparea” variables as parameters.
Finally, you must call the perform() method to help you perform your code instructions.
You must again locate an element “message” shown inside the droparea with “Dropped!” text when the WebElement is dropped. XPath will be used to locate it and then assert that the presented message is correct.
Once the element is dropped, use the assert methods to compare the actual text with the expected text and then use the browser.quit(), close the browser instance.
To learn how to use asserts correctly, follow this blog on using asserts in Selenium WebDriver.
Performing Drag and Drop by Offset in Selenium
Let’s learn how to implement the Selenium drag_and_drop_by_offset() method using Python.
Here, we will use the Selenium click command to drag a WebElement in the DOM to a specific target offset. This involves using the drag_and_drop_by_offset(element, xoffset, yoffset) method of ActionChains.
To better understand the workings of the drag_and_drop_by_offset(element, xoffset, yoffset) method, let’s take a test scenario to demonstrate its functionality.
Test Scenario:
|
You can view the screenshot of the page below, where we will automate drag and drop by offset action.
Code Implementation:
Below is the code implementation of the above test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
from selenium.webdriver.common.by import By from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Key" gridUrl = "hub.lambdatest.com/wd/hub" lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/drag-and-drop-demo") #locating the element draggable = browser.find_element(By.XPATH, value="//p[normalize-space()='Drag me to my target']") # create action chain object actions = ActionChains(browser) actions.drag_and_drop_by_offset(draggable, 80, 30) actions.perform() #asserting that when dropping, "Dropped!" is shown inside the droparea message = browser.find_element(By.XPATH, value="//div[@id='droppable']/p") assert message.text == "Dropped!" #closing the browser browser.quit() |
Code Walkthrough:
In this test scenario, we must again locate the draggable WebElement. We can use XPath again and store the element in the “draggable” variable.
To drag and drop the element by offset, we should call the drag_and_drop_by_offset method, passing the “draggable” variables and the xoffset and yoffset as parameters. In this scenario, we will use the 80 and 30 offsets.
Finally, you must call the perform() method to help you perform your code instructions.
We will again locate an element “message” shown inside the droparea with “Dropped!” text when the WebElement is dropped. We can again use XPath to locate it and then assert that the presented message is correct.
Once the element is dropped, use the assert methods to compare the actual text with the expected text and then use the browser.quit(), close the browser instance.
Performing Move by Offset in Selenium
Let’s learn how to implement the Selenium move_by_offset() method using Python.
Here, we will use the Selenium click command to move the mouse by offsetting the current mouse position. This involves using the move_by_offset(xoffset, yoffset) method of ActionChains.
To better understand the workings of the drag_and_drop_by_offset(element, xoffset, yoffset) method, let’s take a test scenario to demonstrate its functionality.
Test Scenario:
|
You can view the screenshot of the page below, where we will automate move by offset action.
Code Implementation:
Below is the code implementation of the above test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Key" gridUrl = "hub.lambdatest.com/wd/hub" lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/hover-demo") # create action chain object actions = ActionChains(browser) actions.move_by_offset(617, 593) actions.perform() #closing the browser browser.quit() |
Code Walkthrough:
To move the mouse cursor to a specific offset position, you must call the move_by_offset() method, passing the two offset values. In this example, we will use 617 to offset and 593 to offset. Call the perform() method to help you perform your code instructions.
Use the assert methods to compare the actual text with the expected text and then use the browser.quit(), close the browser instance.
Performing Move to Element in Selenium
Let’s learn how to implement the Selenium move_to_element() method using Python.
Here we will use the Selenium click command to move the mouse to a WebElement in the DOM. This involves using the move_to_element(element) method of ActionChains.
To better understand the workings of the move_to_element(element) method, let’s take a test scenario to demonstrate its functionality.
Test Scenario:
|
You can view the screenshot of the page below, where we will automate the move to element action.
Code Implementation:
Below is the code implementation of the above test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
from selenium.webdriver.common.by import By from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Key" gridUrl = "hub.lambdatest.com/wd/hub" lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/hover-demo") #locating the element first_image = browser.find_element(By.XPATH, value="//div[@class='s__column m-15']//img") # create action chain object actions = ActionChains(browser) actions.move_to_element(first_image) actions.perform() from time import sleep sleep(10) #asserting that when hoving, "Hover" is shown below the image message = browser.find_element(By.XPATH, value="//div[@class='s__column m-15']//p") assert message.text == "Hover" #closing the browser browser.quit() |
Code Walkthrough:
In this test scenario, you must locate the first image on the page after configuring the capabilities to access the page. You can use XPath again and store the element in the “first_image” variable.
To move the mouse cursor to the first image, we should call the move_to_element() method, passing the “first_image” variable as a parameter. Finally, you must call the perform() method to help you perform your code instructions.
We will locate an element called “message”, shown below the image with “Hover” text when the mouse is on the image. You can again use XPath to locate it and then assert that the presented message is correct.
Then, use the assert methods to compare the actual text with the expected text and then use the browser.quit(), close the browser instance.
Performing Move to Element With Offset in Selenium
Let’s learn how to implement the Selenium move_to_element_with_offset() method using Python.
Here, we will use the Selenium click commands to perform mouse movement by offsetting the specified WebElement in the DOM. This involves using the move_to_elemenet_with_offset(element, xoffset, yoffset) method of ActionChains.
To better understand the workings of the move_to_elemenet_with_offset(element, xoffset, yoffset) method, let’s take a test scenario to demonstrate its functionality.
Test Scenario:
|
You can view the screenshot of the page below, where we will automate moving to the element with offset action.
Code Implementation:
Below is the code implementation of the above test scenario.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
from selenium.webdriver.common.by import By from selenium import webdriver from selenium.webdriver.common.action_chains import ActionChains #lambdatest setup and opening the desired website username = "Your LambdaTest Username" accessToken = "Your LambdaTest Access Key" gridUrl = "hub.lambdatest.com/wd/hub" lt_options = { "user" : username, "accessKey" : accessToken, "build" : "your build name", "name" : "your test name", "platformName" : "Windows 11", "browserName" : "Chrome", "browserVersion" : "latest", "selenium_version": "latest" } web_driver = webdriver.ChromeOptions() options = web_driver options.set_capability('LT:Options', lt_options) url = "https://"+username+":"+accessToken+"@"+gridUrl browser = webdriver.Remote( command_executor=url, options=options ) browser.maximize_window() browser.get("https://www.lambdatest.com/selenium-playground/hover-demo") #locating the element first_image = browser.find_element(By.XPATH, value="//div[@class='s__column m-15']//img") # create action chain object actions = ActionChains(browser) actions.move_to_element_with_offset(first_image, 100, 200) actions.perform() from time import sleep sleep(10) #asserting that when hoving, "Hover" is shown below the image message = browser.find_element(By.XPATH, value="//div[@class='s__column m-15']//p") assert message.text == "Hover" #closing the browser browser.quit() |
Code Walkthrough:
In this test scenario, we must locate the first image on the page. We can use XPath again and store the element in the “first_image” variable.
Then, to move the mouse cursor to the first image, you must call the move_to_element_with_offset() method, passing the “first_image” variable as a parameter and the two offset values. In this example, we have used 100 to xoffset and 200 to yoffset.
Finally, you must call the perform() method to help you perform your code instructions.
We will again locate an element “message” below the image with “Hover” text when the mouse is on the image. We can again use XPath to locate it and then assert that the presented message is correct.
Then, use the assert methods to compare the actual text with the expected text and then use the browser.quit(), close the browser instance.
Generally, to run each of the tests above, use the following command
python filename.py
Once you run the above command, you will see the result in the console if the console is as shown below:
This means the test was run successfully. You can access the LambdaTest Dashboard page to see the execution steps and watch the video record of the script running.
To learn more about other Selenium methods around keyword and mouse actions, follow this blog on Actions class in Selenium and get detailed insights.
You can also Subscribe to the LambdaTest YouTube Channel and stay updated with the latest tutorials around Selenium testing, Selenium with Java, and more.
Conclusion
We learned how to automate Selenium click commands using Python. We discussed the benefits of using Python and Selenium for test automation, introduced the ActionChains library, and explained how it facilitates mouse actions. We also covered the setup process for configuring the required environments, including running automation tests on a cloud Selenium Grid using the LambdaTest platform.
Additionally, we covered the basics of Python web automation, such as opening a webpage in Chrome. Finally, we demonstrated how to automate mouse actions with Selenium and Python through various test scenarios.
Frequently Asked Questions (FAQs)
How do you perform mouse actions in Selenium Python?
The simplest way to perform a mouse action is using the corresponding ActionChains method. These methods are: click(), double_click(), drag_and_drop(), get_attribute(), get_current_url(), get_title(), is_element_present(), move_to_element(), moveToElementWithId(), moveToElementWithText() and sendKeys().
Can Selenium move the mouse?
The Actions class in Selenium WebDriver enables us to move the mouse pointer to a specific location or element. To create an object of the actions class, we have to use the WebDriver instance created by the webdriver.Create()
method. The MoveToElement()
method in the actions class takes the locator object as a parameter and moves it to that specific location/element. We can move one or more locations/elements using this method.
Can Selenium click buttons?
There are a variety of web page components that one can access while using the Selenium web driver. These include buttons, scroll bars, hyperlinks, etc.
Got Questions? Drop them on LambdaTest Community. Visit now
Related Articles
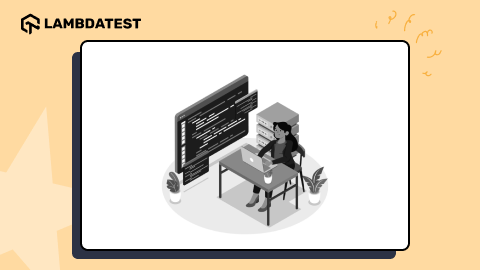
10 Biggest Remote Testing Challenges And How To Resolve [2025]
Praveen Mishra
February 21, 2025
468814 Views
15 Min Read
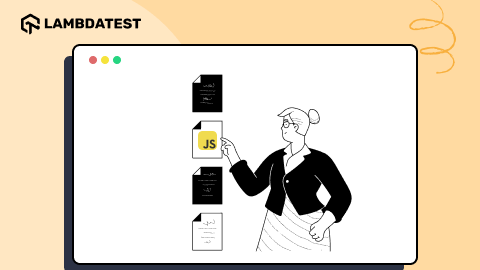
13 JavaScript Testing Best Practices You Should Know [2025]
Arnab Roy Chowdhury
February 20, 2025
263702 Views
14 Min Read
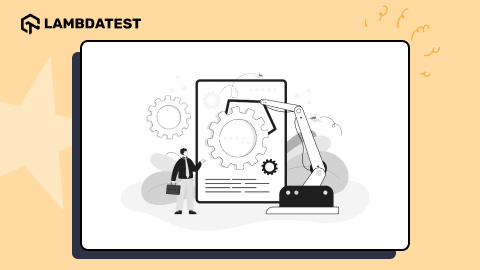
Top Resources To Learn Automation Testing In 2025
Akash Agarwal
112759 Views
13 Min Read
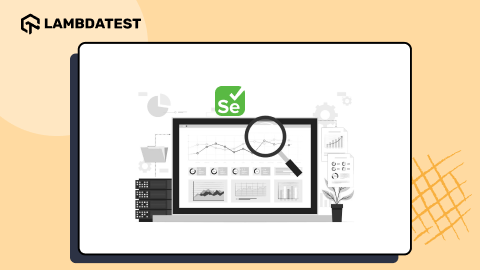
9 Best Reporting Tools for Selenium [2025]
Himanshu Sheth
February 19, 2025
174260 Views
18 Min Read
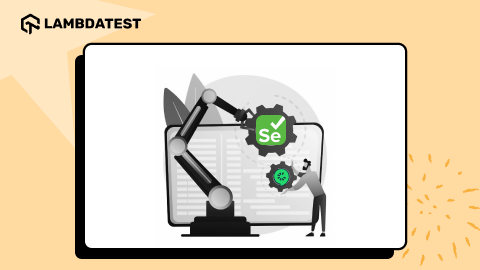
Top 6 Cucumber Best Practices for Selenium Automation [2025]
Harshit Paul
154007 Views
14 Min Read
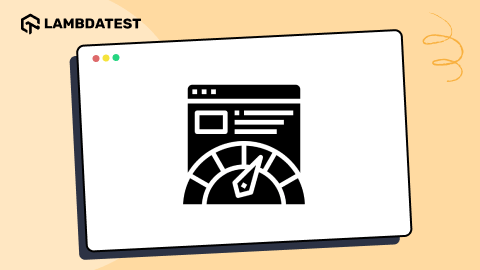
Top 11 Performance Testing Tools for 2025
Akash Nagpal
February 18, 2025
76773 Views
17 Min Read